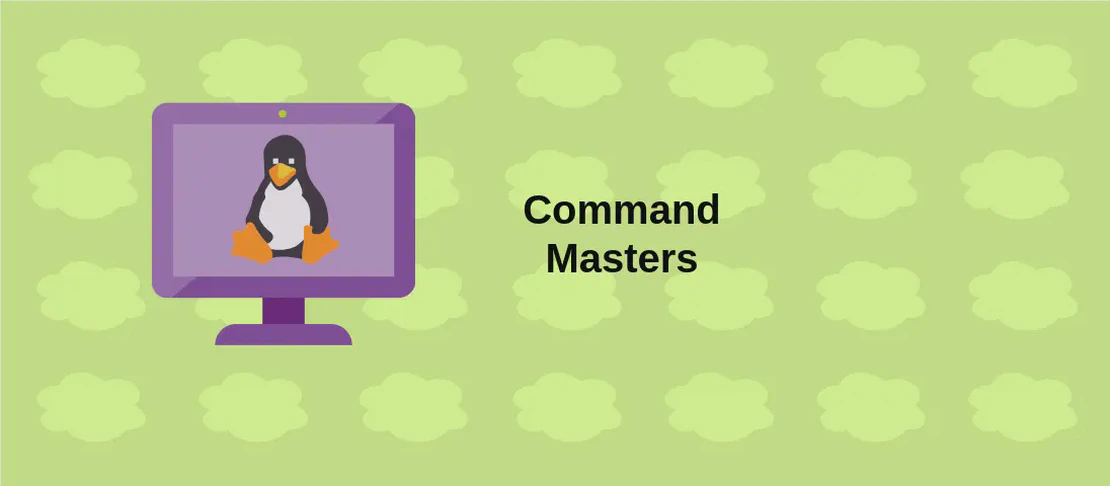
How to use the command 'uvicorn' (with examples)
uvicorn is a command-line utility for running Python web applications that follow the ASGI specification. It is designed to be fast and efficiently handle multiple concurrent connections. The command allows users to run Python web apps, specify the host and port, enable live reloading, configure the number of worker processes, and run the app over HTTPS.
Use case 1: Run Python web app
Code:
uvicorn import.path:app_object
Motivation: The command is used to run a Python web app. By specifying the import path of the app module and the app object, uvicorn starts the ASGI server and serves the app on the default host and port (localhost:8000).
Explanation:
import.path
: The import path of the Python module that contains the app object.app_object
: The name of the app object within the module.
Example output:
INFO: Uvicorn running on http://localhost:8000 (Press CTRL+C to quit)
INFO: Started reloader process [12345]
INFO: Started server process [67890]
Use case 2: Listen on port 8080 on localhost
Code:
uvicorn --host localhost --port 8080 import.path:app_object
Motivation: This use case specifies the host and port on which the Python web app should listen. By setting the host to “localhost” and port to 8080, the app can be accessed locally on a different port than the default (8000).
Explanation:
--host localhost
: Specify the network interface or hostname to bind to (in this case, “localhost”).--port 8080
: Specify the port number to listen on (in this case, 8080).import.path:app_object
: The import path of the Python module that contains the app object.
Example output:
INFO: Uvicorn running on http://localhost:8080 (Press CTRL+C to quit)
INFO: Started reloader process [12345]
INFO: Started server process [67890]
Use case 3: Turn on live reload
Code:
uvicorn --reload import.path:app_object
Motivation: Live reload enables automatic reloading of the web app whenever changes are made to the source code. This is useful during development to see immediate updates without manually restarting the server.
Explanation:
--reload
: Enable automatic reloading of the web app when changes are detected.import.path:app_object
: The import path of the Python module that contains the app object.
Example output:
INFO: Uvicorn running on http://localhost:8000 (Press CTRL+C to quit)
INFO: Started reloader process [12345]
INFO: Started server process [67890]
Use case 4: Use 4 worker processes for handling requests
Code:
uvicorn --workers 4 import.path:app_object
Motivation: By default, uvicorn uses a single worker process to handle requests. This use case specifies the number of worker processes to be used, which can help in scaling the application for concurrent connections and handling high traffic.
Explanation:
--workers 4
: Specifies the number of worker processes to launch for handling requests (in this case, 4).import.path:app_object
: The import path of the Python module that contains the app object.
Example output:
INFO: Uvicorn running on http://localhost:8000 (Press CTRL+C to quit)
INFO: Started reloader process [12345]
INFO: Started server process [67890]
Use case 5: Run app over HTTPS
Code:
uvicorn --ssl-certfile cert.pem --ssl-keyfile key.pem import.path:app_object
Motivation: Running the app over HTTPS provides encryption and secure transmission of data. This use case allows users to specify the SSL certificate and key files for enabling HTTPS.
Explanation:
--ssl-certfile cert.pem
: Specify the path to the SSL certificate file.--ssl-keyfile key.pem
: Specify the path to the SSL private key file.import.path:app_object
: The import path of the Python module that contains the app object.
Example output:
INFO: Uvicorn running on https://localhost:8000 (Press CTRL+C to quit)
INFO: Started reloader process [12345]
INFO: Started server process [67890]
Conclusion:
The uvicorn
command is a versatile tool for running Python web apps following the ASGI specification. It allows for fine-grained control over the server’s configuration, from specifying the host and port to enabling live reloading, scaling the number of worker processes, and running the app over HTTPS. By leveraging these use cases, developers can effectively deploy and manage Python web applications with ease.