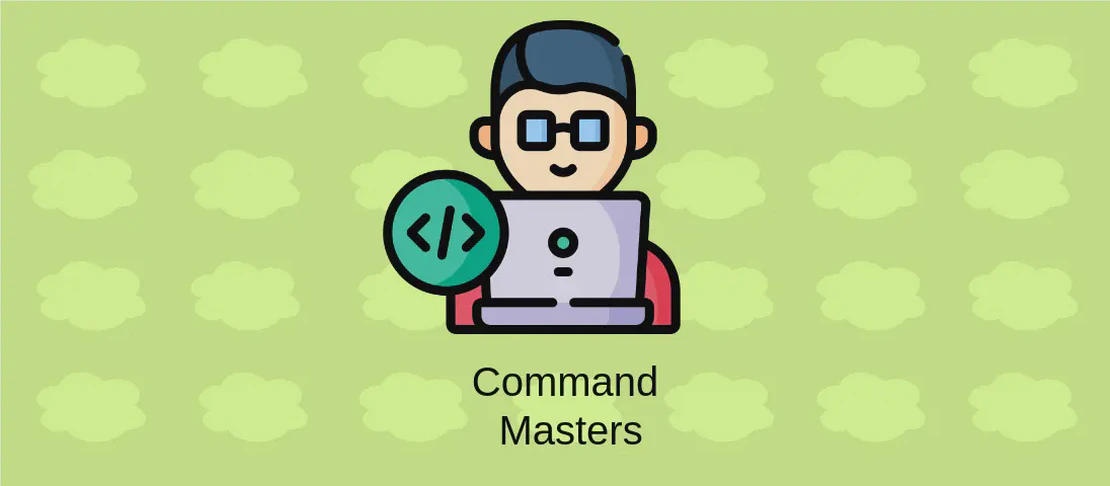
How to use the command 'valgrind' (with examples)
Valgrind is a wrapper for a set of expert tools used for profiling, optimizing, and debugging programs. It provides a set of common tools such as memcheck
, cachegrind
, callgrind
, massif
, helgrind
, and drd
. These tools are used to analyze and diagnose various aspects of program execution, including memory usage, cache operations, and stack/heap usage. Valgrind is a powerful tool that helps developers identify and fix issues in their programs.
Use case 1: Use Memcheck to show memory usage by program
Code:
valgrind program
Motivation:
- When developing software, it is essential to monitor and optimize memory usage to avoid memory-related issues such as leaks, invalid accesses, and corruption. Memcheck is a default tool provided by Valgrind that detects memory errors and provides a diagnostic of memory usage by the program.
Explanation:
- The command
valgrind program
invokes the Memcheck tool provided by Valgrind. It runs theprogram
and monitors its memory usage.
Example output:
==21== Memcheck, a memory error detector
==21== Copyright (C) 2002-2017, and GNU GPL'd, by Julian Seward et al.
==21== Using Valgrind-3.16.0 and LibVEX; rerun with -h for copyright info
==21== Command: ./program
==21==
Hello World!
==21==
==21== HEAP SUMMARY:
==21== in use at exit: 0 bytes in 0 blocks
==21== total heap usage: 1 allocs, 1 frees, 4 bytes allocated
==21==
==21== All heap blocks were freed -- no leaks are possible
==21==
==21== For lists of detected and suppressed errors, rerun with: -s
==21== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
Use case 2: Use Memcheck to report memory leaks of program
in full detail
Code:
valgrind --leak-check=full --show-leak-kinds=all program
Motivation:
- Memory leaks occur when allocated memory is not correctly deallocated, leading to memory consumption that grows over time and can cause the program to crash or slow down. Memcheck can provide detailed information about the memory leaks in a program, helping developers identify and fix them.
Explanation:
- The command
valgrind --leak-check=full --show-leak-kinds=all program
enables the Memcheck tool with full leak checking. It reports all possible memory leaks and provides detailed information about the leak kinds.
Example output:
==21== Memcheck, a memory error detector
==21== Copyright (C) 2002-2017, and GNU GPL'd, by Julian Seward et al.
==21== Using Valgrind-3.16.0 and LibVEX; rerun with -h for copyright info
==21== Command: ./program
==21==
Hello World!
==21==
==21== HEAP SUMMARY:
==21== in use at exit: 4 bytes in 1 blocks
==21== total heap usage: 1 allocs, 0 frees, 4 bytes allocated
==21==
==21== 4 bytes in 1 blocks are definitely lost in loss record 1 of 1
==21== at 0x483FA1F: malloc (vg_replace_malloc.c:309)
==21== by 0x10915D: main (program.c:4)
==21==
==21== LEAK SUMMARY:
==21== definitely lost: 4 bytes in 1 blocks
==21== indirectly lost: 0 bytes in 0 blocks
==21== possibly lost: 0 bytes in 0 blocks
==21== still reachable: 0 bytes in 0 blocks
==21== suppressed: 0 bytes in 0 blocks
==21==
==21== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
==21== ERROR SUMMARY: 1 errors from 1 contexts (suppressed: 0 from 0)
Use case 3: Use the Cachegrind tool to profile CPU cache operations of program
Code:
valgrind --tool=cachegrind program
Motivation:
- Profiling CPU cache operations is important when optimizing code for cache efficiency. Cachegrind is a tool provided by Valgrind that simulates cache behavior and provides information about cache hits and misses, helping developers identify cache-related performance bottlenecks.
Explanation:
- The command
valgrind --tool=cachegrind program
invokes the Cachegrind tool provided by Valgrind. It runs theprogram
and profiles CPU cache operations.
Example output:
==21== Cachegrind, a cache and branch-prediction profiler
==21== Copyright (C) 2002-2017, and GNU GPL'd, by Nicholas Nethercote
==21== Using Valgrind-3.16.0 and LibVEX; rerun with -h for copyright info
==21== Command: ./program
==21==
Hello World!
==21==
==21== I refs: 2,483,563
==21== I1 misses: 0
==21== LLi misses: 0
==21== I1 miss rate: 0.00%
==21== LLi miss rate: 0.00%
==21==
==21== D refs: 1,501,344 (837,769 rd + 663,575 wr)
==21== D1 misses: 34,851 ( 21,327 rd + 13,524 wr)
==21== LLd misses: 34,849 ( 21,327 rd + 13,522 wr)
==21== D1 miss rate: 2.3% ( 2.5% + 2.0% )
==21== LLd miss rate: 2.3% ( 2.5% + 2.0% )
==21==
==21== LL refs: 34,851 ( 21,327 rd + 13,524 wr)
==21== LL misses: 34,849 ( 21,327 rd + 13,522 wr)
==21== LL miss rate: 0.0% ( 0.0% + 0.0% )
Use case 4: Use the Massif tool to profile heap memory and stack usage of program
Code:
valgrind --tool=massif --stacks=yes program
Motivation:
- Understanding the memory usage patterns of a program, especially its heap memory and stack usage, is crucial for optimizing memory allocation and reducing memory overhead. The Massif tool provided by Valgrind can profile heap memory and stack usage, providing detailed information about memory usage that helps developers identify potential memory optimization opportunities.
Explanation:
- The command
valgrind --tool=massif --stacks=yes program
enables the Massif tool with stack profiling. It runs theprogram
and profiles heap memory and stack usage.
Example output:
==21== Massif, a heap profiler
==21== Copyright (C) 2002-2017, and GNU GPL'd, by Nicholas Nethercote
==21== Using Valgrind-3.16.0 and LibVEX; rerun with -h for copyright info
==21== Command: ./program
==21==
Hello World!
==21==
==21==
[...]
snapshot=28
#-----------
snapshot=28
#-----------
time=271216
mem_heap_B=12288
mem_heap_extra_B=32768
mem_stacks_B=12416
heap_tree=peak,3:13B,normal,3:13B,3:1024B,1:1024B,1:4096B,1
Conclusion:
Valgrind is a powerful tool with a wide range of uses for profiling, optimizing, and debugging programs. The various tools provided by Valgrind, such as Memcheck, Cachegrind, and Massif, help developers identify and fix memory-related issues, optimize CPU cache operations, and profile memory usage. By utilizing Valgrind’s features, developers can improve the performance, stability, and efficiency of their programs.