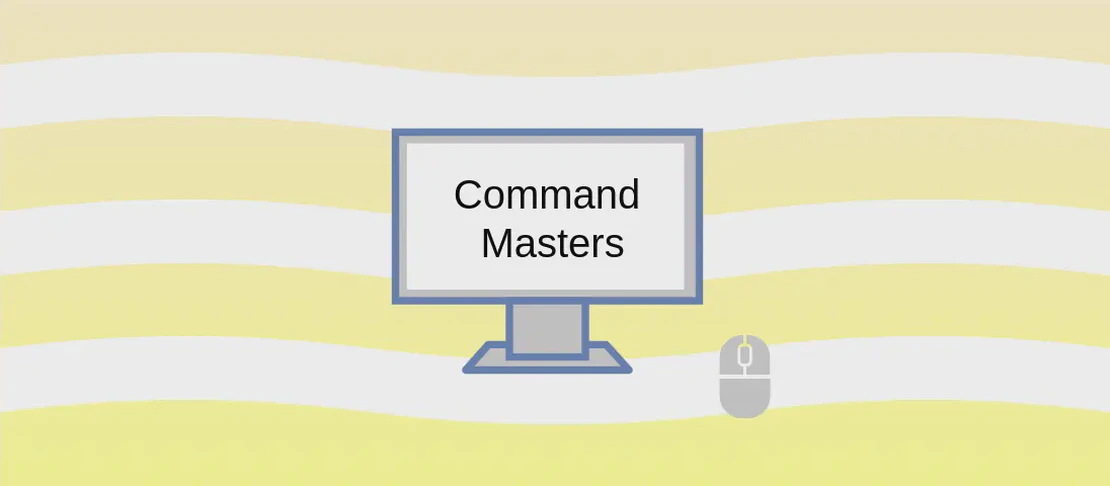
How to use the command `vcpkg` (with examples)
vcpkg
is a package manager specifically designed for managing C/C++ libraries. It simplifies the process of acquiring and integrating libraries into your C/C++ projects. Unlike some package managers, packages managed by vcpkg
are not directly installed in your system environment. Instead, they are installed in a custom directory, and you configure your build system, such as CMake, to utilize these packages. This makes it easier to manage dependencies without polluting your global environment and helps maintain consistent build environments across different systems.
Use case 1: Build and add package libcurl
to the vcpkg
environment
Code:
vcpkg install curl
Motivation:
The motivation for using this command is to add the popular libcurl
library to your project. libcurl
is a versatile and lightweight library for transferring data with URLs. It’s often used for downloading files, initiating HTTP requests, and interacting with various internet protocols. Having libcurl
as a dependency may be essential for developers working on applications that require robust and flexible data transfer capabilities.
Explanation:
vcpkg
: This initiates thevcpkg
package manager.install
: The install command tellsvcpkg
to fetch and build the specified library.curl
: This is the name of thelibcurl
package to be installed.
Example Output:
The following packages will be built and installed:
curl[core]:x86-windows
* openssl[core]:x86-windows
* zlib[core]:x86-windows
Additional packages (*) will be installed to complete this operation.
Starting package 1/3: zlib:x86-windows
...
Use case 2: Build and add zlib
using the emscripten
toolchain
Code:
vcpkg install --triplet=wasm32-emscripten zlib
Motivation:
This example demonstrates how to build and add the zlib
library using the emscripten
toolchain, which is particularly useful for developers targeting WebAssembly environments. zlib
is a widely-used data compression library, and its availability in a WebAssembly format enables web applications to handle compressed data efficiently. By using the emscripten
toolchain, developers can ensure that their applications can support browsers and other environments where WebAssembly is relevant.
Explanation:
vcpkg
: Indicates the use of thevcpkg
package manager.install
: Command to instructvcpkg
to fetch and build the library.--triplet=wasm32-emscripten
: This flag specifies the target architecture and toolchain.wasm32-emscripten
denotes building for WebAssembly using the Emscripten compiler.zlib
: The library name to be installed.
Example Output:
The following packages will be built and installed:
zlib[core]:wasm32-emscripten
...
Use case 3: Search for a package
Code:
vcpkg search pkg_name
Motivation:
When a developer needs to find specific libraries or check available versions within the vcpkg
package manager, this command is extremely helpful. It provides a quick way to determine if a particular library is available for use in a project, aiding in assessing potential dependencies and planning development efforts.
Explanation:
vcpkg
: Defines the use of thevcpkg
package manager.search
: Command to search the indexes of available packages withinvcpkg
.pkg_name
: Placeholder representing the package name being searched for. Replace it with the actual name of the library.
Example Output:
curl 7.75.0#1 A library for transferring data with URLs.
zlib 1.2.11#9 A compression library
...
Use case 4: Configure a CMake project to use vcpkg
packages
Code:
cmake -B build -DCMAKE_TOOLCHAIN_FILE=path/to/vcpkg_install_directory/scripts/buildsystems/vcpkg.cmake
Motivation:
This use case illustrates how to configure a CMake project to automatically discover and use libraries installed via vcpkg
. When you want to streamline the integration of third-party libraries in your build system, correctly configuring CMake with vcpkg
ensures that dependencies are properly linked and available to your project, simplifying dependency management and improving productivity.
Explanation:
cmake
: This is the command to invoke the CMake build system.-B build
: This argument specifies the build directory, where all generated build files will be stored.-DCMAKE_TOOLCHAIN_FILE=...
: This is a CMake specific option to set the path to the toolchain file. The toolchain file provided byvcpkg
instructs CMake on how to find and usevcpkg
libraries, ensuring seamless integration into your project. Replacepath/to/vcpkg_install_directory
with the actual path to your vcpkg installation directory.
Example Output:
-- The C compiler identification is GNU 9.3.0
-- The CXX compiler identification is GNU 9.3.0
-- Detecting C compiler ABI info
-- Detecting C compiler ABI info - done
-- Check for working C compiler: /usr/bin/cc - skipped
...
Conclusion:
vcpkg
is a powerful tool for managing C/C++ libraries, offering a flexible and clean way to handle package dependencies. By understanding the different use cases and examples, developers can more effectively incorporate and manage libraries in their projects. From installing popular libraries like libcurl
to configuring projects with CMake, vcpkg
streamlines the setup and maintenance of dependencies, paving the way for more efficient and maintainable C/C++ development workflows.