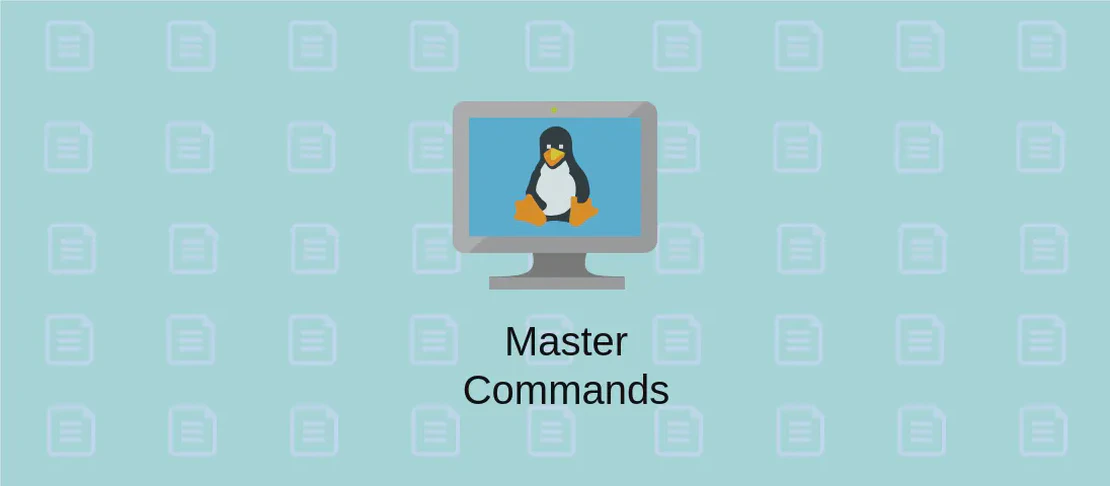
How to Use the Command 'venv' in Python (with Examples)
Python’s venv
module is an essential tool for developers who need to manage dependencies and package versions for different projects independently. It enables the creation of isolated Python environments, ensuring that libraries required for one project won’t interfere with others. This isolation is crucial when projects need differing versions of libraries or Python itself.
Create a Python Virtual Environment
Code:
python -m venv path/to/virtual_environment
Motivation: Creating a virtual environment is the foundational step when starting a new Python project or when you need an isolated environment for experimenting with new libraries. By separating environments, you can avoid dependency conflicts and can easily reproduce the same environment setup across different systems. This step ensures that each project remains self-contained, making collaboration and project sharing more manageable.
Explanation:
python
: This is the command to execute Python. Depending on your installation, you might need to usepython3
.-m
: This flag tells Python to run the module as a script.venv
: This is the module being called to execute the command. It stands for “virtual environment.”path/to/virtual_environment
: This is the directory where the virtual environment will be created. You should replace this with your desired path.
Example Output:
Upon execution, a directory will be created at the specified path. It will contain directories like bin/
(Linux/macOS) or Scripts/
(Windows), which hold the executables, and lib/
or Lib/
, which contains a copy of the Python interpreter and supporting files necessary to run Python applications within the environment. You won’t often see explicit output on the command line unless there’s an error, but you can confirm its creation by navigating to the specified directory.
Activate the Virtual Environment (Linux and macOS)
Code:
source path/to/virtual_environment/bin/activate
Motivation:
To work within the isolated environment, you must activate the virtual environment. Activation modifies your shell’s environment variables, so commands like python
and pip
use the executables and libraries in the virtual environment rather than the system-wide Python installation. This ensures that you are working with the correct dependencies and configurations specific to your project.
Explanation:
source
: A shell command used to execute the file in the current shell. This is essential to modify the environment variables of the current shell.path/to/virtual_environment/bin/activate
: The activation script specific to your configured virtual environment. This script alters the shell environment to use the virtual environment’s Python and pip.
Example Output:
Once activated, your command line prompt will change to show the name of your virtual environment, like (your_env_name)user@machine:~$
, indicating you’re operating within the virtual environment. Python-related commands will now use the appropriate executables and libraries designated by the environment.
Activate the Virtual Environment (Windows)
Code:
path\to\virtual_environment\Scripts\activate.bat
Motivation: The usage of virtual environments in Windows follows the same logic as in Linux and macOS. Activating the environment ensures that your command prompt Python operations utilize the isolated environment, providing confidence that your project’s dependencies won’t clash with any globally installed packages.
Explanation:
path\to\virtual_environment\Scripts\activate.bat
: The batch file specific to the generated virtual environment. Unlike Unix-style operating systems, Windows uses batch scripts to execute activation commands that set the environment to use isolated executables and libraries of the virtual environment.
Example Output:
After execution, the command prompt will reflect the active virtual environment, such as (your_env_name) C:\Users\YourUser>
to show that the virtual environment is active, and subsequent Python executions within this prompt will respect the isolated environment rules.
Deactivate the Virtual Environment
Code:
deactivate
Motivation: When you’re finished working in a particular project or want to switch to other environments, you must deactivate the current virtual environment to return to the system-wide Python installation. This ensures that your terminal environment returns to its prior state without any custom paths or dynamic modifications made by the activation script.
Explanation:
deactivate
: A command available in an active virtual environment used to undo all changes made to the current shell environment. It essentially ‘unsets’ any environment variables changed during the activation.
Example Output:
Executing this command will remove the environment name from your prompt, reverting it to its original state, e.g., user@machine:~$
. This indicates that you’ve exited the isolated virtual environment and are back in the global environment.
Conclusion:
Using Python’s venv
is indispensable for effective Python project management, especially when juggling multiple projects with distinct dependencies. Whether it’s setting up a new environment, activating it for use, or deactivating it after, each step ensures smooth development without the hassle of dependency conflicts, leading to more robust and manageable codebases.