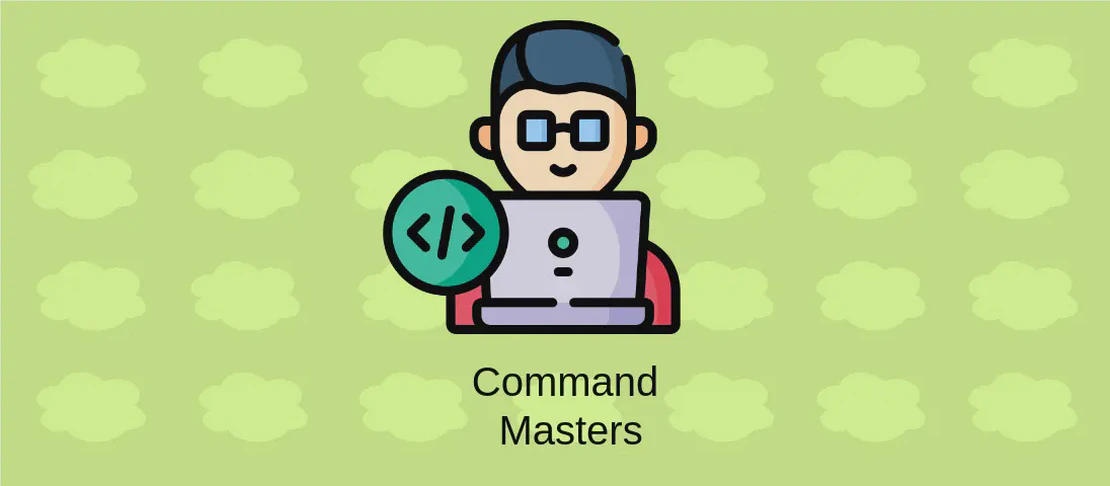
How to Use the Verilator Command (with Examples)
Verilator is a free and open-source tool used primarily for the conversion of Verilog and SystemVerilog hardware description languages (HDL) into C++ or SystemC models. Once converted, these models can be executed after a suitable compilation process. Verilator is particularly useful for simulating digital circuits at high speeds, providing a C++-based simulation environment. This feature proves invaluable for the design and verification of hardware systems, allowing hardware engineers and developers to efficiently test and validate their designs. For more information, you can visit the official guide here: Verilator Guide .
Use case 1: Build a specific C project in the current directory
Code:
verilator --binary --build-jobs 0 -Wall path/to/source.v
Motivation:
This use case focuses on building a specific C project. In the realm of hardware design verification, it’s often necessary to convert and compile HDL models into binary files that can be executed to observe and validate behavior. By executing this command, users leverage Verilator’s unique ability to handle these tasks efficiently in a UNIX-like environment, simplifying the C++ binary creation process.
Explanation:
verilator
: The main command used for initiating the Verilator tool.--binary
: This argument specifies that the tool should generate a binary executable from the input Verilog source file.--build-jobs 0
: This specifies the number of parallel jobs to use when building the project. A value of0
means that Verilator automatically selects the number of jobs based on available resources, maximizing efficiency.-Wall
: A safety flag that enables all warning messages during compilation, critical for catching potential issues early in the process.path/to/source.v
: This represents the path to the Verilog source file that is to be converted and compiled into a binary executable.
Example Output:
INFO: Running Verilator
INFO: Building binary from source.v
INFO: Compilation successful — Binary created.
Use case 2: Create a C++ executable in a specific folder
Code:
verilator --cc --exe --build --build-jobs 0 -Wall path/to/source.cpp path/to/output.v
Motivation:
This use case pertains to the seamless conversion and compilation of C++ executables that integrate HDL models. This process enhances interoperability by allowing the Verilog model to be compiled alongside existing C++ codebases, a highly effective strategy for developing larger, more complex hardware-software systems.
Explanation:
--cc
: Instructs Verilator to translate the Verilog source into C++.--exe
: This flag indicates that an executable should be created.--build
: Combines the translation and build process, embedding it all into one efficient command.--build-jobs 0
: Sets the build to take advantage of multiprocessing capabilities, automatically adjusting the tasks based on the system’s core availability.-Wall
: Provides warnings to ensure the build process remains free of semantic errors or potential pitfalls.path/to/source.cpp
: Specifies the path to additional C++ source files, allowing for integration with the Verilog design.path/to/output.v
: Pertains to the Verilog model to be converted to C++ within the specified environment.
Example Output:
INFO: Converting Verilog model to C++
INFO: Compiling C++ executable from source.cpp and output.v
INFO: C++ executable successfully created.
Use case 3: Perform linting over a code in the current directory
Code:
verilator --lint-only -Wall
Motivation:
Linting serves as a crucial initial step in the development cycle, especially in hardware description languages, where subtle mistakes can lead to significant functional errors. By performing a lint check with Verilator, developers effectively identify syntactic and stylistic inconsistencies in their HDL code, ensuring a high standard of code quality before proceeding to subsequent stages like synthesis or simulation.
Explanation:
--lint-only
: Commands Verilator to run a lint check on the existing Verilog files without initiating the synthesis process.-Wall
: Ensures comprehensive warning generation, serving as a proactive tool for code improvement by listing all detected issues for review.
Example Output:
INFO: Linting Verilog files
WARNING: assignment in conditional expression on line 23
INFO: 0 errors, 1 warning.
Use case 4: Create XML output about the design
Code:
verilator --xml-output -Wall path/to/output.xml
Motivation:
Generating a detailed XML output of a hardware design allows designers to document and interface within automated design workflows effectively. This structured format is beneficial for interoperability with other tools, facilitating further processing and analysis of components like module instances, file hierarchy, and utilized data types—essentials for sophisticated digital design work.
Explanation:
--xml-output
: Directs Verilator to create an XML document representing the design overview, capturing all essential aspects of the HDL such as hierarchy and logic.-Wall
: Preserves the vigilance concerning warnings during this conversion, maintaining awareness of potential issues.path/to/output.xml
: Specifies the file path where the generated XML file is saved, enabling users to decide its destination and accessibility.
Example Output:
INFO: Generating XML output for the design
INFO: XML output written to path/to/output.xml
Conclusion:
As demonstrated in these use cases, Verilator serves as a powerful tool for multiple stages of the HDL design and verification process. Its capabilities span linting for error-checking, compiling for C++ execution, and design documentation in XML format. With the use of comprehensive flags and commands, developers can harness these functionalities to streamline their workflow, ensuring their digital designs are efficient, accurate, and readily integrable into larger systems.