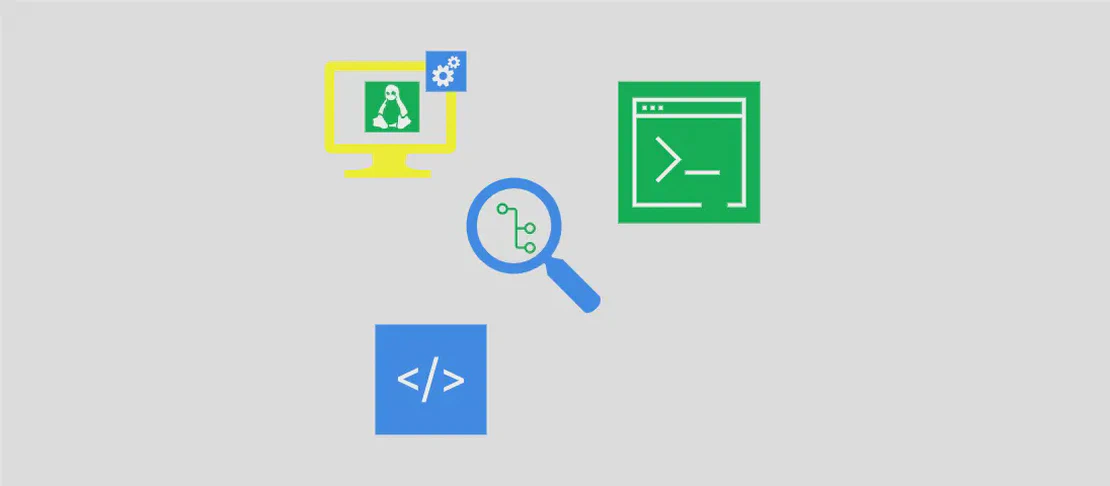
How to use the command 'vim' (with examples)
Vim, also known as Vi IMproved, is a versatile and powerful command-line text editor that provides users with extensive tools for text manipulation and software development. Known for its efficiency, Vim can be utilized in various modes, each designed to handle different types of tasks, from writing and editing text to executing complex commands. Vim’s ability to be customized extensively makes it a popular choice among programmers and system administrators. The text editor is accessible across various operating systems, and its key feature is the modal nature of editing, allowing users to switch between different modes for inserting text, command execution, and visual text selection.
Use case 1: Open a file
Code:
vim path/to/file
Motivation:
Opening a file with Vim is the quintessential action for any user wanting to edit text. This command is particularly useful for developers and system admins who work on configuration files, scripts, or lengthy documents. Vim’s efficiency stems from its speed, which allows you to open large files without performance degradation.
Explanation:
vim
: This invokes the Vim text editor program from the command line.path/to/file
: This specifies the path to the file you wish to open. This can be a relative path from your current directory, or an absolute path from the root directory.
Example output:
When the command is executed, Vim launches in normal mode, displaying the contents of the specified file, ready for editing or viewing.
Use case 2: Open a file at a specified line number
Code:
vim +line_number path/to/file
Motivation:
This command is particularly useful when you need to quickly navigate to a specific section of a file, such as when debugging code or reviewing logs. If an error message or a tool pinpoints a problematic line, this command helps in jumping directly to the location without manually scrolling through the file.
Explanation:
vim
: Initiates the Vim editor.+line_number
: This tells Vim to directly navigate to the specified line number upon opening the file.path/to/file
: Specifies the file path you want to open.
Example output:
Vim opens with the cursor positioned at the beginning of the specified line, allowing immediate interaction with the desired section of text.
Use case 3: View Vim’s help manual
Code:
:help<Enter>
Motivation:
The help manual of Vim is an essential resource for both new users and experienced ones who are looking to master Vim or learn about new features. The help system is comprehensive and provides detailed explanations along with practical examples.
Explanation:
:help
: In normal mode, typing:
begins a command prompt;help
followed by pressing<Enter>
loads the extensive help documentation within Vim.
Example output:
A buffer opens within Vim displaying detailed documentation about the usage of Vim, containing links to various topics, descriptions, and examples.
Use case 4: Save and quit the current buffer
Code:
<Esc>ZZ|<Esc>:x<Enter>|<Esc>:wq<Enter>
Motivation:
Efficient saving and exiting from a file are critical operations, particularly when editing is finished or changes need to be preserved immediately. It enhances workflow dynamics by providing different methods to achieve the same goal, allowing choice in user preference.
Explanation:
<Esc>ZZ
: From normal mode,ZZ
saves the file and exits Vim.<Esc>:x
: Moves into command mode from where:x
commands Vim to save the file if changes were made and then exit.<Esc>:wq
: Another method through which:w
writes changes to the file and:q
quits Vim, all in a single command.
Example output:
The file saves successfully, and Vim exits to the shell, confirming that all changes are recorded.
Use case 5: Enter normal mode and undo the last operation
Code:
<Esc>u
Motivation:
Undoing previous changes is a routine necessity while editing documents, whether to correct mistakes or backtrack on modifications that no longer align with the objective. Vim provides an intuitive, powerful undo feature that enhances its user-friendliness.
Explanation:
<Esc>
: Ensures you are in normal mode, which is required to execute commands likeu
.u
: The undo command in Vim, reverting the last change made.
Example output:
Vim undoes the last operation, and the document’s state reverts to how it was before the latest change.
Use case 6: Search for a pattern in the file
Code:
/search_pattern<Enter>
Motivation:
Searching for text patterns allows users to quickly locate specific content in a text file, a feature frequently used in coding to find functions, variables, or documentation in lengthy scripts.
Explanation:
/
: Initiates search mode in Vim.search_pattern
: The string or regular expression pattern you want to find.<Enter>
: Executes the search, taking you to the next occurrence of the pattern.
Example output:
The cursor moves to the first occurrence of the pattern in the text. Every subsequent press of n
moves the cursor to the next match, while N
moves it to the previous one.
Use case 7: Perform a regular expression substitution in the whole file
Code:
:%s/regular_expression/replacement/g<Enter>
Motivation:
Batch editing operations across a file save significant time, particularly for repetitive changes needed in scripts or documents. This example demonstrates how regular expressions can be applied to substitute text swiftly across an entire document.
Explanation:
:%
: Specifies that the substitution should be performed on all lines in the file.s
: Stands for substitution.regular_expression
: The pattern you want to replace.replacement
: The text that will replace the matched patterns.g
: Stands for ‘global’, indicating that all occurrences in every line should be replaced.
Example output:
The text specified by your regular_expression
is replaced by replacement
throughout the file.
Use case 8: Display the line numbers
Code:
:set nu<Enter>
Motivation:
Displaying line numbers is advantageous for coding since it aids in navigation, debugging, and collaborating on code by providing a common reference point. It makes it easier to track the location of changes or follow instructions.
Explanation:
:set nu
: Engages Vim’s line numbering mode, indicating visually where each line begins relative to the start of the file.
Example output:
Each line in the file is prefixed by its corresponding line number, providing a visual cue for easier navigation.
Conclusion:
Vim’s robust set of features caters to a broad spectrum of text editing needs, from basic file manipulation to complex text processing tasks. By exploring each of these use cases, users can leverage Vim’s full potential to streamline their workflow, enhance productivity, and improve overall editing and coding efficiency.