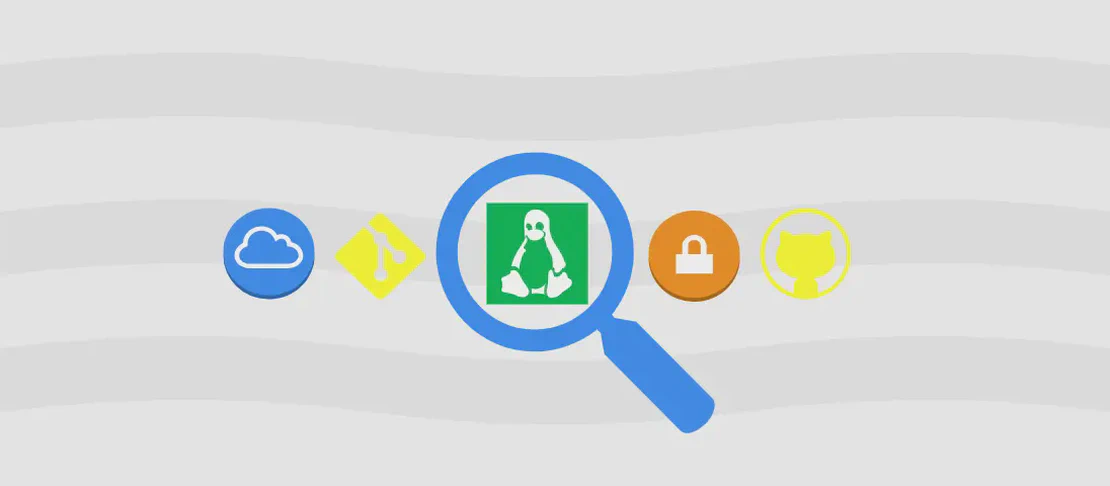
How to use the command 'virtualenv' (with examples)
Virtualenv is a powerful and lightweight tool used in Python programming to create isolated environments for your projects. It helps keep dependencies required by different projects in separate places, thus avoiding conflicts and providing greater control over the software you are developing. By using virtualenv, you can manage package versions effectively without impacting other projects on your system. This is especially useful when managing projects that depend on different libraries or specific versions of the same library.
Create a new environment
Code:
virtualenv path/to/venv
Motivation:
When starting a new Python project or maintaining an existing one, it is crucial to have an isolated environment to manage dependencies. Creating a new virtual environment allows developers to install specific packages required for the project without interfering with other projects on the same machine.
Explanation:
virtualenv
: This is the command used to initiate a new virtual environment.path/to/venv
: This represents the destination path where the virtual environment will be created. You should replace “path/to/venv” with your desired directory location.
Example Output:
When you run this command, a new directory will be created at the specified path, containing all necessary files to maintain the isolated environment, such as ‘bin’, ’lib’, and ‘include’ directories. You will see output indicating the creation of these directories, confirming that your virtual environment is ready for use.
Customize the prompt prefix
Code:
virtualenv --prompt=prompt_prefix path/to/venv
Motivation:
Customizing the prompt prefix is beneficial for developers who manage multiple virtual environments and want a clear visual indication of which environment is currently active. This helps in minimizing errors, such as installing packages in the wrong environment.
Explanation:
--prompt=prompt_prefix
: This flag allows you to define a custom prompt prefix that appears when the environment is activated. It helps denote the environment in the shell prompt.path/to/venv
: This specifies where the virtual environment will be set up, similar to the previous use case.
Example Output:
After activating the environment with a customized prompt, your command line interface will display the specified prompt prefix, making it easier to identify the active environment. The shell prompt might look something like this: (prompt_prefix) user@machine:path/to/your/project$
Use a different version of Python with virtualenv
Code:
virtualenv --python=path/to/pythonbin path/to/venv
Motivation:
As new Python versions are released, developers may need to test their code against different versions of Python. Using a virtual environment with a specified Python interpreter allows you to create an isolated environment for your project with the desired version of Python.
Explanation:
--python=path/to/pythonbin
: This option specifies an alternative Python interpreter for the virtual environment. You should replace “path/to/pythonbin” with the path to the desired Python binary.path/to/venv
: This is the directory where the virtual environment will be created.
Example Output:
Upon running this command, the virtual environment will use the specified Python interpreter. This allows you to use features or syntax specific to that Python version, ensuring compatibility and further enabling thorough testing and development.
Start (select) the environment
Code:
source path/to/venv/bin/activate
Motivation:
Activating a virtual environment is necessary to work within it. This ensures that all Python commands and package installations use the environment’s isolated settings and dependencies, protecting your global Python environment from unintended changes.
Explanation:
source
: This command is used to run the activate script and update the shell environment settings.path/to/venv/bin/activate
: This path points to the activation script within your virtual environment’s directory structure. It switches your shell to operate within the virtual environment.
Example Output:
After activation, your command line will change to show the active virtual environment’s name, often prepended to your shell prompt. This indicator helps remind you that all Python-related operations will affect this environment.
Stop the environment
Code:
deactivate
Motivation:
Once you’ve finished work in a virtual environment, it’s important to deactivate it to return to your default system settings or to prepare for activating a different environment. This helps avoid confusion and maintains system integrity.
Explanation:
deactivate
: This is a simple command used to exit the virtual environment and return to the system’s default Python environment.
Example Output:
Upon running the deactivate
command, the environment’s prompt will disappear, and the shell returns to its default state, no longer associating Python commands with the active virtual environment.
Conclusion:
The virtualenv tool is essential for managing Python project dependencies, allowing developers to work with multiple projects without conflict. By creating isolated environments, customizing prompts, specifying Python versions, and effectively managing activation states, developers gain greater flexibility and organization in their workflows. Understanding these use cases ensures optimal use of virtualenv, providing a solid foundation for any Python project development.