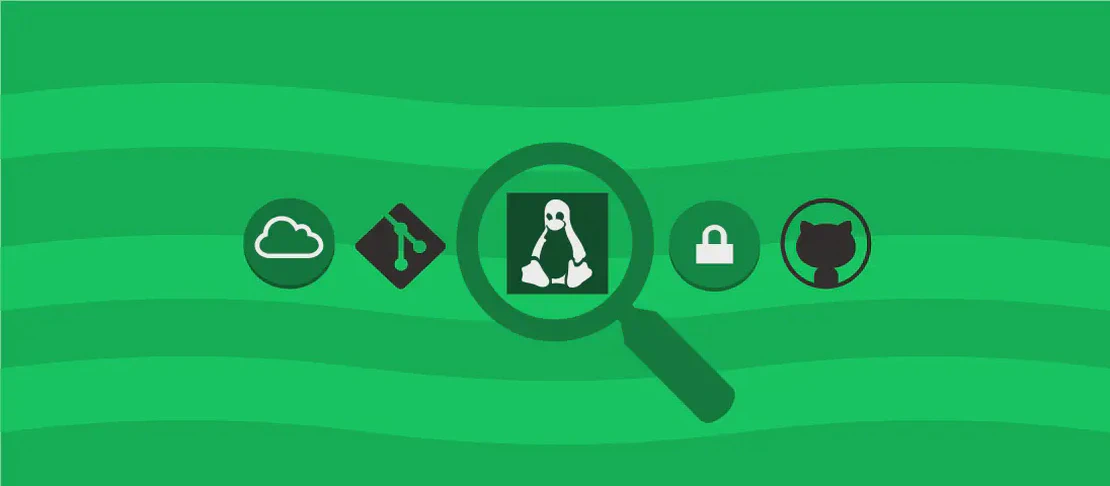
How to Create a Vite Project (with examples)
Vite is a next-generation front-end build tool that significantly improves the speed and efficiency of JavaScript project development. It allows developers to quickly spin up projects using various templates like vanilla JavaScript, Vue, React, Svelte, and more. Vite provides an incredibly fast development server and a highly optimized build for production. This article delves into the different use cases for setting up a Vite project using different package managers like npm, yarn, and pnpm, specifically for a React TypeScript setup.
Use case 1: Setup using npm
6.x
Code:
npm create vite@latest my-react-app --template react-ts
Motivation:
When using npm
version 6.x, which is commonly found in some stable and long-term versions of Node.js, this command allows you to create a new Vite project tailored for React with TypeScript. The motivation behind this is to utilize Vite’s fast development environment to build React applications with TypeScript’s type-checking benefits.
Explanation:
npm create vite@latest
: This initializes a new Vite project using thecreate-vite
package from the latest version available on npm. Thecreate
keyword is the standard way to scaffold new projects in the npm ecosystem.my-react-app
: This is the name assigned to your project. It will create a directory with this name.--template react-ts
: The--template
flag specifies the project template to be used, in this case, a React project with TypeScript.
Example output:
Upon running this command, a directory named my-react-app
will be created, containing all the necessary setup for a Vite-powered React TypeScript project. You will see output indicating the progress of downloading and installing the necessary packages.
Use case 2: Setup using npm
7+, extra double-dash is needed
Code:
npm create vite@latest my-react-app -- --template react-ts
Motivation:
For users who have transitioned to npm
version 7 or higher, an extra double-dash --
is required to separate the arguments passed to the vite
package. This aligns with npm
’s new argument parsing mechanism, ensuring compatibility and correctly passing arguments intended for Vite.
Explanation:
npm create vite@latest
: Similar to the previous use case, this initializes a new Vite project using the latestcreate-vite
package.my-react-app
: The designated project name and directory.--
: This double-dash separates thenpm
command’s core arguments from those intended for the Vite command.--template react-ts
: Tells Vite to scaffold the project as a React app with TypeScript.
Example output:
The output will be similar to the first use case, with the extra double-dash ensuring correct parsing. Expect feedback on the creation of project directories and installation of dependencies.
Use case 3: Setup using yarn
Code:
yarn create vite my-react-app --template react-ts
Motivation:
For those who prefer yarn
, a popular package manager touted for its speed and security, this setup command initiates the creation of a Vite project. The motivation here is the seamless integration with yarn
, providing an efficient and familiar approach to managing JavaScript project dependencies.
Explanation:
yarn create vite
: This initializes a Vite project using thevite
template, similar to the npm command but specifically designed for yarn.my-react-app
: The project will bear this name, establishing a new directory.--template react-ts
: Directs yarn to set up a React project with TypeScript support.
Example output:
The terminal will provide feedback on yarn’s actions, such as fetching packages, setting up the project structure, and ensuring that all dependencies specific to a React TypeScript application are correctly installed.
Use case 4: Setup using pnpm
Code:
pnpm create vite my-react-app --template react-ts
Motivation:
Using pnpm
, known for its efficient handling of disk space and speed, this command sets up a Vite project. Developers motivated by performance and who want to leverage pnpm
’s unique features will find this setup particularly advantageous.
Explanation:
pnpm create vite
: Initializes the Vite project usingpnpm
. This ensures the creation benefits from pnpm’s fast and disk-efficient module management.my-react-app
: The name and root directory of your new project.--template react-ts
: Specifies the template as React with TypeScript, aligning the project with the desired development stack.
Example output:
A clear output will indicate the creation of the project directory, the use of pnpm to install dependencies, and completion notices upon structuring a ready-to-develop environment for your React and TypeScript project.
Conclusion:
Vite offers a blazing-fast environment for modern front-end development, and its setup process is flexible across various package managers like npm, yarn, and pnpm. Utilizing the correct command depending on your package manager can greatly streamline the initial setup of a React and TypeScript project. Each example demonstrates Vite’s versatility and efficiency, making it an ideal choice for developers looking to boost productivity in their JavaScript projects.