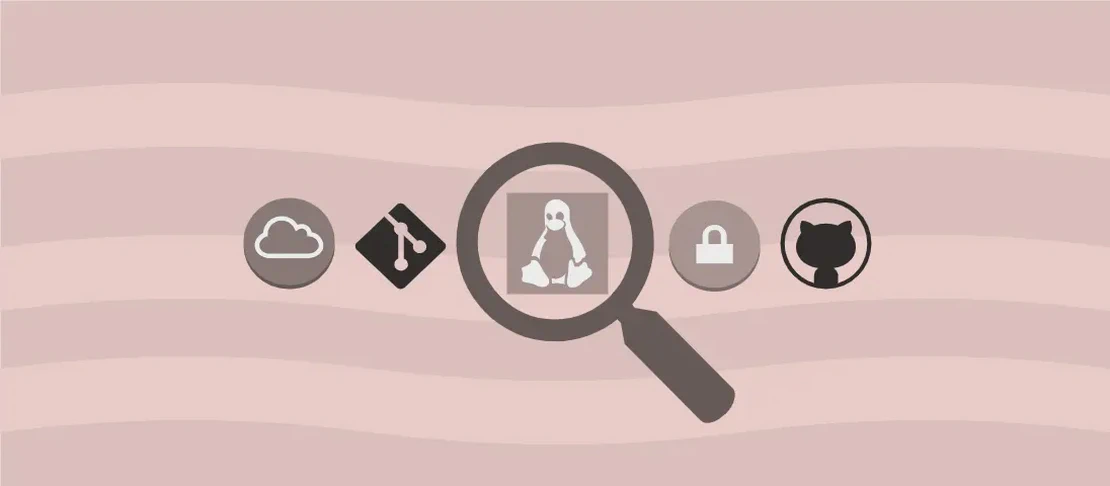
How to use the command 'wait' (with examples)
The wait
command is a utility found in Unix and Unix-like operating systems, used to pause the execution of a script or process until a specified background process has finished. This command is particularly useful in shell scripting, allowing for the synchronization of processes. When a script has initiated multiple background processes, wait
can be employed to ensure these processes complete before the script continues to subsequent operations.
Use case 1: Wait for a process to finish given its process ID (PID) and return its exit status
Code:
wait pid
Motivation:
Suppose you are running a background task in your shell script and you want to ensure that it has finished before you proceed to the next step of your automation script. By using wait
with a specific process ID, you can target a single process and enforce that further execution in your script happens only after this process completes. This is crucial in scenarios where the data or results produced by one task are required for succeeding tasks.
Explanation:
pid
: The process ID of the task whose completion you are waiting for. Every running process in a Unix-like system is assigned a unique PID. Thewait
command, when followed by a PID, instructs the system to hold on proceeding until this specific process concludes its execution. It then returns the exit status of that process, which can be checked to determine if the process completed successfully or encountered an error.
Example Output:
Let’s say you have a backup process running with PID 1234. When it completes, the wait
command might return:
[1234] + Done backup-script.sh
This indicates that the backup process with PID 1234 has finished.
Use case 2: Wait for all processes known to the invoking shell to finish
Code:
wait
Motivation:
In scenarios where a script or an individual has launched multiple processes and it is essential that all these processes are completed before taking further action, using wait
without arguments serves as a blanket statement. This ensures that the shell pauses to verify the termination of all processes it initiated. Such utilization is particularly valuable in large scripts that rely on multiple background tasks to run concurrently, aiming for efficient execution and reduced wait times between dependent operations.
Explanation:
- Omitting any arguments with the
wait
command tells the shell to apply the ‘wait’ to all the background processes that the shell knows about. This is a catch-all statement that ensures every single background task initiated under the shell’s context has been allowed to complete, providing a clean slate for the continuation of the script or any other tasks.
Example Output:
If there were several processes, you might see output as each concludes:
[1] Done data-process.sh
[2] Done cleanup-script.sh
This output signifies that all the relevant background processes launched have successfully completed.
Use case 3: Wait for a job to finish
Code:
wait %N
Motivation:
When working with job control in a shell environment, jobs are tracked using a job number. This form of using wait
is particularly helpful when managing job control interactions through a script or a command line session. It aims to provide specific synchronization when working with job numbers, ensuring that advanced shell features are reliably coordinated and completed before moving forward with additional tasks. This is vital in interactive sessions where jobs are suspended, backgrounded, or managed with the fg
, bg
, or jobs
commands.
Explanation:
%N
: This represents a job number, or a placeholder signifying a job managed through the shell’s job control utility. Job numbers are assigned when a command is run in the background, and you can see them by typingjobs
in the terminal. Usingwait
with a job number ensures that the exact job specified is completed before proceeding, making it a precise tool for job control.
Example Output:
If you are waiting for job number 2, the output might be:
[2]+ Done example-job.sh
This output confirms that the job with the designated number 2 has finished executing.
Conclusion:
The wait
command is an essential component in the Unix/Linux shell environment for managing process synchronization. Whether you need to pause execution until one specific process completes, ensure that all processes finish before moving forward, or keep precise track of jobs in interactive scenarios, wait
provides a robust solution. Mastery of this command can significantly improve the efficiency and reliability of your shell scripts and system management activities.