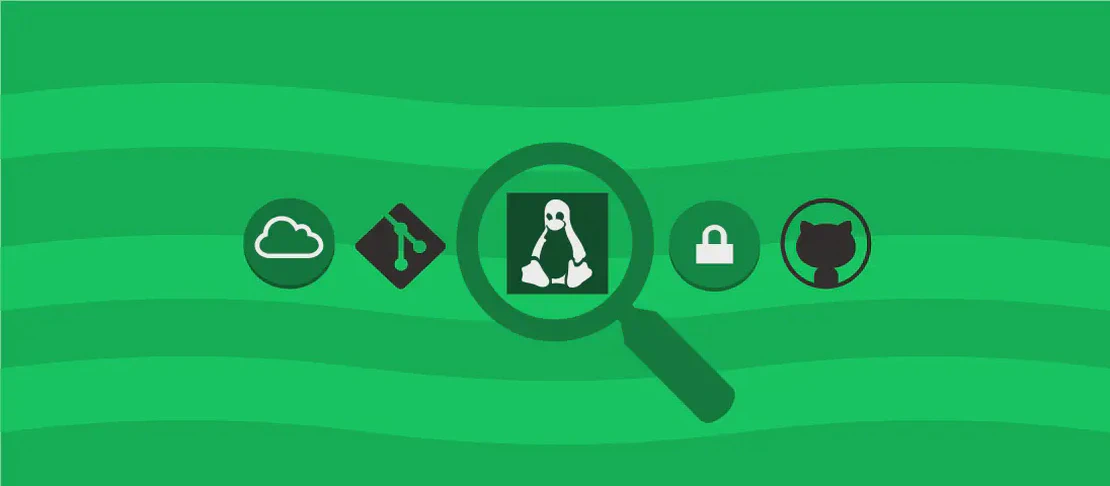
Using the 'Wait-Process' PowerShell Command (with examples)
The PowerShell Wait-Process
command is a useful tool for PowerShell script management, designed to pause script execution until specified processes have completed. It enhances process control, allowing scripts to wait until certain tasks are finished before moving on to the next operation, thus ensuring a smoother and more coordinated execution flow. This can be particularly useful in scenarios where dependencies or resource allocations require that particular processes fully terminate before the initiation of subsequent commands or scripts.
Use case 1: Stop a process and wait
Code:
Stop-Process -Id process_id; Wait-Process -Id process_id
Motivation for use:
Sometimes, a process that is currently running can cause conflicts or resource contentions that need resolution before subsequent code is executed. For instance, you might need to stop an application, such as a development environment, before updating files that it uses. In such cases, the ability to halt a process and ensure its termination before proceeding is vital. This command sequence ensures that the process is terminated and handled completely, without any lingering instances that might cause unexpected issues.
Explanation:
Stop-Process
: This command sends a termination signal to a process based on its-Id
. By stopping a process explicitly, you ensure that the process does not consume further resources or interfere with subsequent operations.-Id process_id
: The-Id
parameter is used to specify the identification number of the process you wish to stop. Each running process is assigned a unique ID by the operating system.;
: This semicolon is simply used to concatenate commands in PowerShell, allowing both to be executed sequentially.Wait-Process
: This part of the command waits for the process (identified by the same ID) to terminate completely, ensuring that it is fully stopped before any proceeding commands are executed.
Example Output:
When this code is executed, it stops the specified process and pauses until it is fully terminated. While there is no visual output from Wait-Process
, its function ensures the process is accurately tracked until completion. Users might only notice a pause until the command sees the process has been successfully concluded.
Use case 2: Wait for processes for a specified time
Code:
Wait-Process -Name process_name -Timeout 30
Motivation for use:
There are situations where you don’t necessarily need to or can’t stop a process manually, but you still require that it be finished or reach a stopped state before executing further actions. An example could be waiting for a database backup process to complete before starting a cleanup job. This command line waits for an assigned period, after which the script continues regardless of whether the process has stopped, ensuring that resources are engaged efficiently within a defined timeframe.
Explanation:
Wait-Process
: Again, this is a waiting command for a specified process to terminate before moving forward in the script.-Name process_name
: The-Name
parameter allows you to specify processes by their executable names, which can often be more intuitive and user-friendly than memorizing process IDs. For example, processes likenotepad
orchrome
can be referenced directly.-Timeout 30
: The-Timeout
parameter indicates the maximum number of seconds the command will wait for the process to stop. After 30 seconds, the command will move on, regardless of whether the process is still running. This ensures that the script isn’t indefinitely delayed by a process that isn’t terminating as expected.
Example Output:
This command will run and wait for up to 30 seconds for the specified process to complete. If the process stops anytime within this period, the rest of the script continues immediately. If, on the other hand, the process continues beyond 30 seconds, the script will proceed as well, without a direct console output indicating the wait termination.
Conclusion:
The Wait-Process
command is an essential tool for process synchronization in PowerShell scripting, providing mechanisms to either wait for expected completions or handle processes with a built-in timeout, thus allowing scripts to operate both patiently and efficiently. These use cases demonstrate how to manage processes smoothly, avoiding bottlenecks and potential conflicts within your scripting workflows by structuring dependencies precisely.