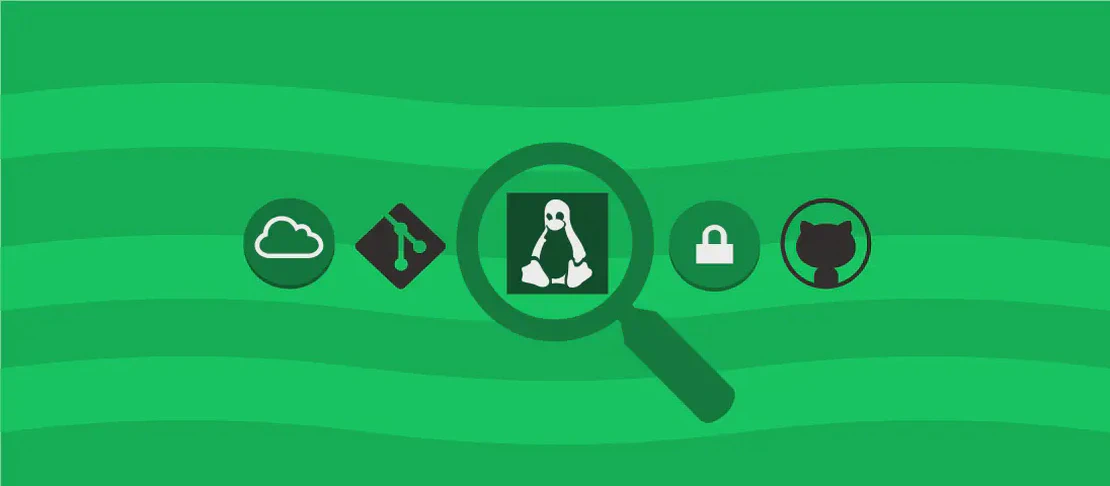
How to use the command 'waitress-serve' (with examples)
Waitress is a popular, production-quality pure-Python WSGI server designed to host Python web applications. It provides developers with a simple command to serve their applications with flexibility for various networking configurations. The waitress-serve
command is often chosen due to its ease of use, robustness, and support for both synchronous and asynchronous web applications. It is particularly valuable when you need a reliable solution without the complexity of more extensive systems.
Run a Python web app
Code:
waitress-serve import.path:wsgi_func
Motivation:
This use case represents the most straightforward deployment of a Python web application, where you have a WSGI application callable ready for hosting. It demonstrates how easily one can transition from development to a live running instance using Python’s intrinsic functionality.
Explanation:
import.path:wsgi_func
: This argument specifies the module and callable within the module that represents the WSGI application. Replaceimport.path
with the actual Python import path of the module andwsgi_func
with the function or callable object implementing the WSGI interface.
Example output:
Serving on http://0.0.0.0:8080
Listen on port 8080 on localhost
Code:
waitress-serve --listen=localhost:8080 import.path:wsgi_func
Motivation:
Often, applications need to be confined to specific addresses or ports, particularly for development and testing environments, where you don’t need the visibility of external interfaces. Hosting on localhost
ensures security by limiting access to only the machine hosting the application.
Explanation:
--listen=localhost:8080
: Specifies that Waitress should listen for requests on thelocalhost
address at port8080
. This confines the server to only handle requests originating from the same machine.
Example output:
Serving on http://localhost:8080
Start waitress on a Unix socket
Code:
waitress-serve --unix-socket=path/to/socket import.path:wsgi_func
Motivation:
Deploying an application using a Unix socket can be advantageous for efficient inter-process communication on the same host. This setup is often used in environments where performance is critical, and where external TCP/IP connections are not required.
Explanation:
--unix-socket=path/to/socket
: Directs the waitress server to bind to a Unix domain socket instead of a network address. Replacepath/to/socket
with the actual filesystem path where the socket file should be created.
Example output:
Serving on unix:/path/to/socket
Use 4 threads to process requests
Code:
waitress-serve --threads=4 import.path:wsgi_func
Motivation:
Threading is a means of optimizing server responsiveness, especially under multiple concurrent connections. By increasing the number of threads, the server can handle more simultaneous requests, which increases throughput and enhances performance, particularly in high-load scenarios.
Explanation:
--threads=4
: Configures Waitress to use 4 threads for handling incoming requests. More threads can handle more simultaneous requests, but keep server load and resources in mind when configuring.
Example output:
Serving on http://0.0.0.0:8080 with 4 threads
Call a factory method that returns a WSGI object
Code:
waitress-serve --call import.path.wsgi_factory
Motivation:
Some applications might encapsulate their setup logic within a factory function to create WSGI application instances. This is particularly useful when the application requires configuration during instantiation or multiple instances with different initial properties.
Explanation:
--call
: Instructs Waitress to call a specified factory method in place of directly using an application callable. This allows the flexibility to execute setup code before returning the WSGI application.import.path.wsgi_factory
: The import path to the factory function that will be called to obtain the WSGI callable.
Example output:
Using factory function from import.path.wsgi_factory
Serving on http://0.0.0.0:8080
Use the HTTPS URL scheme
Code:
waitress-serve --url-scheme=https import.path:wsgi_func
Motivation:
In today’s web environments, using HTTPS is often mandatory due to security requirements. This example shows how to adjust the URL scheme for applications that natively support security measures or when integrated into larger systems that provide HTTPS offloading.
Explanation:
--url-scheme=https
: Modifies the URL scheme used by Waitress to HTTPS. This is significant for applications or middleware relying on URL scheme detection to work correctly.
Example output:
Serving on https://0.0.0.0:8080
Conclusion:
The waitress-serve
command is an essential tool for deploying Python web applications. Its ease of use and flexibility make it an excellent choice for developers looking to deploy applications in various environments, from local development setups to more robust production scenarios. The command can be tailored through its numerous options to meet specific requirements, enhancing the application’s adaptability to different networking and performance conditions.