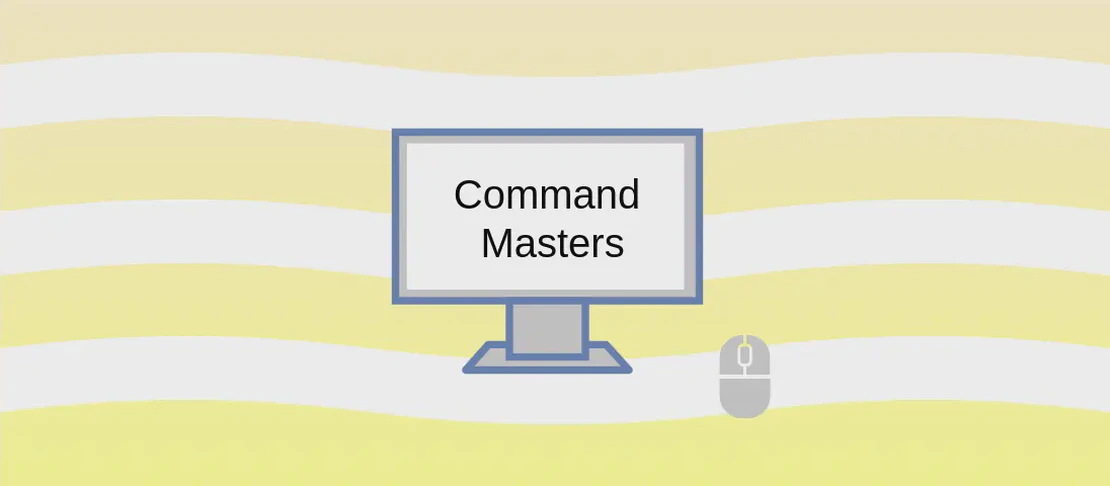
How to Optimize WebAssembly Binaries Using 'wasm-opt' (with examples)
The wasm-opt
tool is a powerful command-line utility used for optimizing WebAssembly binary files. Created as part of the Binaryen project, wasm-opt
aims to reduce the size of WebAssembly files, optimize their performance, and facilitate an efficient execution flow. By using various optimization levels and options, developers can enhance the execution speed of WebAssembly modules and decrease their memory footprint, making them ideal for web applications with performance constraints. Below are detailed examples of how you can utilize this tool effectively.
Use case 1: Apply default optimizations and write to a given file
Code:
wasm-opt -O input.wasm -o output.wasm
Motivation:
This simple yet effective use case is best suited when you want to optimize a WebAssembly file using default configuration settings. The default optimizations tend to strike a balanced trade-off between improving execution speed and maintaining an acceptable transformation time. This is ideal in cases where you want quick improvements without diving into granular optimization settings.
Explanation:
-O
: This option enables the default optimizations ofwasm-opt
, which include both size and performance improvements.input.wasm
: This parameter specifies the input WebAssembly file to be optimized.-o output.wasm
: The-o
flag specifies the output file, which will be the optimized version of the WebAssembly module. The original input remains unchanged.
Example Output:
Upon executing the above command, you can expect the output.wasm
file to be about 20-30% smaller and faster, based on the complexity of the original file. Actual metrics can vary depending on original code intricacies and wasm-opt
version enhancements.
Use case 2: Apply all optimizations and write to a given file (takes more time, but generates optimal code)
Code:
wasm-opt -O4 input.wasm -o output.wasm
Motivation:
When maximum optimization of a WebAssembly binary is paramount, perhaps for production-level deployment, running comprehensive optimization using -O4
is recommended. Although this mode could take considerably longer to execute due to the depth of transformations applied, it results in highly efficient code that executes faster and is usually smaller in size.
Explanation:
-O4
: This is a more aggressive optimization level that attempts every possible transformation to reduce size and improve performance, effectively using all available optimization techniques.input.wasm
: The WebAssembly file you wish to optimize.-o output.wasm
: It directs the optimized output to a designated file, keeping the original file intact while saving the optimized form asoutput.wasm
.
Example Output:
The output file output.wasm
might show substantial performance gains and size reduction compared to a file optimized with a lower level, depending on the original binary’s content. The processing time, however, could be noticeably longer.
Use case 3: Optimize a file for size
Code:
wasm-opt -Oz input.wasm -o output.wasm
Motivation:
WebAssembly often powers client-side applications, where download size directly impacts load times and bandwidth usage. The -Oz
option is purposefully utilized to minimize the size of a WebAssembly file, prioritizing reduction in file size over execution speed enhancements. This is useful in contexts where limited bandwidth or fast transmission is critical.
Explanation:
-Oz
: This flag instructswasm-opt
to focus specifically on reducing the size of the binary, even at the expense of some performance optimizations.input.wasm
: The file to be optimized.-o output.wasm
: Specifies the output file path for the size-optimized WebAssembly module.
Example Output:
Executing this will yield an output file, output.wasm
, that is smaller in size than both the -O
and potentially even the -O4
optimized versions. This size reduction is primarily beneficial for faster downloads and reduced server load.
Use case 4: Print the textual representation of the binary to console
Code:
wasm-opt input.wasm --print
Motivation:
Understanding the internal structure of a WebAssembly module can be crucial for debugging and further manual optimization. The --print
flag allows developers to inspect the binary in a human-readable textual format. This can be particularly beneficial when tracking down specific functions or debugging particular sections of the code.
Explanation:
input.wasm
: The WebAssembly binary file you wish to examine.--print
: This command option converts the binary input to a textual format and prints it to the console, allowing for human-readable inspection.
Example Output:
Upon execution, the terminal will display the WebAssembly module’s structure, including functions and sections in a readable text format that resembles a simplified version of the WebAssembly Text Format (WAT).
Conclusion:
The wasm-opt
tool offers a spectrum of utilities that cater to various optimization needs, from simple performance enhancements to comprehensive size reductions and in-depth examination. By employing the tool’s multiple command options, developers can finely tune their WebAssembly binaries to achieve desired performance benchmarks, enhance load times, and optimize bandwidth usage on the web.