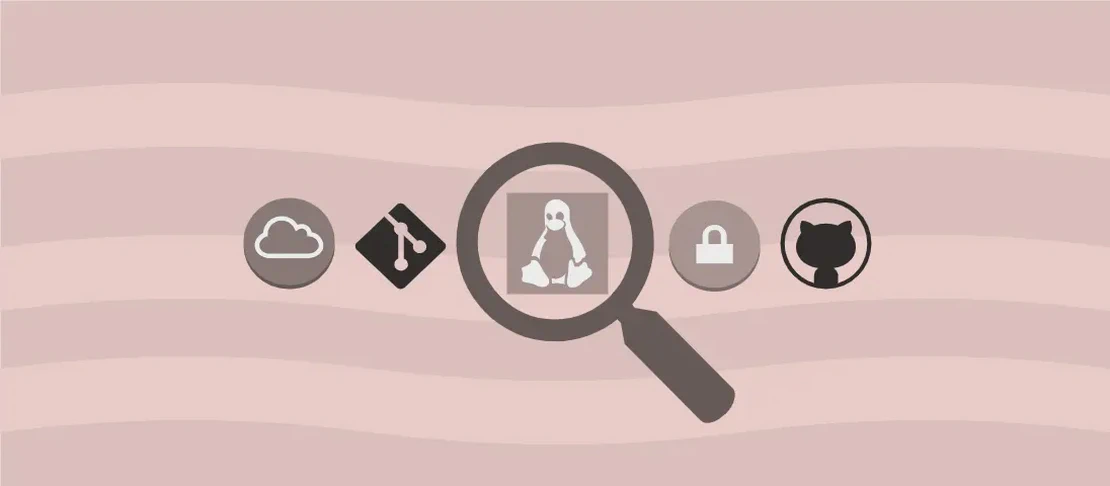
How to use the command 'wasm2c' (with examples)
The wasm2c
command is a powerful utility provided by the WebAssembly Binary Toolkit (WABT). Its primary function is to convert files from the WebAssembly binary format into C source files and headers, allowing developers to work with WebAssembly in environments or situations where C code is preferred or required. This can be particularly useful in cases where integration with existing C codebases is necessary or when exploring the behavior of WebAssembly modules in a more traditional C development setting.
Use case 1: Convert a file to a C source file and header and display it to the console
Code:
wasm2c file.wasm
Motivation for using this example:
Converting a WebAssembly binary to C source code and displaying it on the console can be incredibly useful for developers who want to quickly inspect and understand the structure and content of a .wasm
file. This method allows for an immediate, quick-view without the need for additional file handling, granting users a way to preview and analyze the C representation without altering their directory or files.
Explanation for every argument given in the command:
wasm2c
: This is the command that initiates the process of converting a WebAssembly binary file (.wasm
) into C code. It leverages the tools provided within the WABT toolkit.file.wasm
: This is the input file, a WebAssembly binary that you wish to convert. This must be a valid.wasm
file that you have already created or obtained.
Example output:
After executing this command, the resulting C source code, along with its corresponding declarations and header information, will be displayed on the console. This output will typically include various C structs and functions corresponding to the WebAssembly operations, providing an interface that the rest of your C application can interact with.
Use case 2: Write the output to a given file (file.h
gets additionally generated)
Code:
wasm2c file.wasm -o file.c
Motivation for using this example:
For developers who wish to utilize the output C code in another project or for deeper inspection and modification, saving the converted code into a separate file is essential. This use case allows the source code to be easily integrated, compiled, and utilized within other C-based projects. Additionally, generating a header file ensures that function interfaces and necessary declarations are easily accessible and usable.
Explanation for every argument given in the command:
wasm2c
: Initiates the conversion of the WebAssembly file into C language source code.file.wasm
: Specifies the input file for the conversion process, which should be in the standard WebAssembly binary format.-o file.c
: The-o
flag specifies the output file name where the converted C source code should be written. Accompanying this, thewasm2c
command automatically generates a corresponding header file (file.h
), which contains necessary prototypes and interfaces.
Example output:
Executing this command writes the translated C code into a file named file.c
and creates a file.h
file. Opening file.c
will reveal the comprehensive C representation of the original WebAssembly binary, organized into functions and data structures. Simultaneously, file.h
will contain declarations, making it easier to reference functions and data within other parts of the project.
Conclusion:
The wasm2c
command is an invaluable tool for developers working with WebAssembly, particularly when integration or further development within a C environment is required. By providing both immediate, on-console output and the ability to save files for extensive work, this utility supports varied workflows and developer needs. With these examples, users can effectively harness the power of wasm2c
for both quick analysis and deeper project integration.