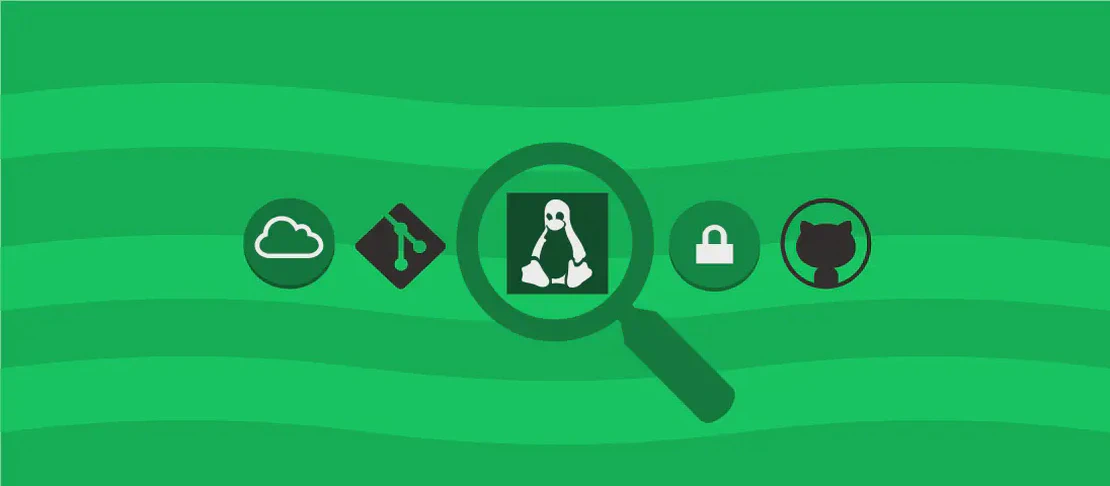
How to use the command 'webpack' (with examples)
Webpack is a static module bundler for modern JavaScript applications. It takes modules with dependencies and generates static assets representing those modules. This tool is highly beneficial for web developers, enabling them to efficiently manage and bundle files like JavaScript and CSS into a single file or multiple files, improving load times and enhancing performance. For more detailed information, visit webpack.js.org .
Use case 1: Create a single output file from an entry point file
Code:
webpack app.js bundle.js
Motivation:
Creating a single output file from an entry point file is the most basic use case of webpack. When you are developing a web application, your project might have multiple JavaScript files working together. For performance optimization, it is beneficial to bundle all these files into one, reducing the number of HTTP requests that the browser has to make to load your application. This use case is crucial for minimizing the loading time and ensuring all dependencies are managed efficiently.
Explanation:
webpack
: This calls the webpack command line tool to start the bundling process.app.js
: This is the entry point for the webpack. It specifies the initial file or module from which the dependency graph will be created.bundle.js
: This is the output file name where all bundled code will be saved. It consolidates all the components of your application into a single JavaScript file.
Example Output:
Upon running the command, you will see messages in your terminal indicating the progress of the bundling process. A potential output might look like:
Hash: a1b2c3d4e5
Version: webpack 5.4.0
Time: 1200ms
Built at: 10/12/2023 10:00:00 AM
Asset Size Chunks Chunk Names
bundle.js 1.5 MiB main [emitted] [big] main
Entrypoint main [big] = bundle.js
Use case 2: Load CSS files too from the JavaScript file
Code:
webpack app.js bundle.js --module-bind 'css=css'
Motivation:
Often, while developing web applications, CSS is used alongside JavaScript. Integrating CSS files into your JavaScript modules simplifies the management of your styles and script resources as it reduces the number of files to bracket together for your application. This is extremely vital for projects aiming for cleaner codebases and streamlined build processes, as it allows developers to manage styles and scripts through a unified entry point.
Explanation:
webpack
: Initiates the webpack tool.app.js
: Serves as the entry point for identifying the dependency graph for both JavaScript and CSS files.bundle.js
: The output file that will include both the JavaScript and the necessary styles packaged together.--module-bind 'css=css'
: This flag tells webpack to additionally process CSS files found in JavaScript. It specifies that all CSS imports (import 'style.css'
) should be handled with the CSS loader, ensuring that stylesheets are included in the bundled output.
Example Output:
You might see an output similar to the following, showing the progress and confirmations of including CSS in the build:
Hash: e6f7g8h9i0
Version: webpack 5.4.0
Time: 1300ms
Built at: 10/12/2023 10:15:00 AM
Asset Size Chunks Chunk Names
bundle.js 1.6 MiB main [emitted] [big] main
Entrypoint main [big] = bundle.js
Use case 3: Pass a configuration file and show compilation progress
Code:
webpack --config webpack.config.js --progress
Motivation:
For larger projects with more complex requirements, managing all the tasks and configurations directly in the command line becomes difficult and error-prone. Having a separate configuration file allows you to define complex configurations including multiple entry points, output options, rules for using different loaders, plugins, and optimizations. Tracking the compilation progress is also a useful feature during development as it provides a visual cue on the build process and timing.
Explanation:
webpack
: Starts the webpack bundle operation.--config webpack.config.js
: This flag tells webpack to read a specific configuration file. This file may specify multiple entry points, output files, module rules, plugins, and more, ensuring all project-specific rules are followed and consistent across builds.--progress
: Displays the build progress in the terminal. It’s particularly useful for long build times as it provides visual feedback about the percentage of the build completed, allowing developers to anticipate how long more the build will take.
Example Output:
Running this will inform you of which stage the compilation process is in and its progress:
[webpack.Progress] 75% ... - building modules
...
[webpack.Progress] 100% ... - done
Use case 4: Automatically recompile on changes to project files
Code:
webpack --watch app.js bundle.js
Motivation:
In a development workflow, it’s highly efficient to have changes automatically detected and compiled in real-time. The --watch
mode is extremely valuable as it increases productivity by reducing the manual steps a developer must perform when updating code. Every change in files will instantly update the bundled files, eliminating the need to manually trigger a compile process after every modification.
Explanation:
webpack
: Starts the bundle process.--watch
: Enables webpack to “watch” for file changes. This keeps webpack active, waiting for any modifications in the source files.app.js
: Acts as the entry point file for the webpack process.bundle.js
: Specifies the output file which is automatically updated upon any source file changes.
Example Output:
When operating with the --watch
feature, webpack remains active in your terminal, displaying messages such as:
webpack is watching the files...
Hash: j1k2l3m4n5
Version: webpack 5.4.0
Time: 400ms
Built at: 10/12/2023 10:30:30 AM
Asset Size Chunks Chunk Names
bundle.js 1.5 MiB main [emitted] main
Whenever a file is changed and saved, you’ll see similar notifications indicating that the re-bundling has occured.
Conclusion:
In conclusion, webpack is a powerful tool for bundling JavaScript and other assets for web projects. Its flexibility allows developers to define custom workflows and automate repetitive tasks efficiently, simplifying the development process while enhancing application performance. With the pervasiveness of JavaScript in modern web applications, understanding and employing webpack can significantly aid in producing well-structured, performant, and scalable codebases.