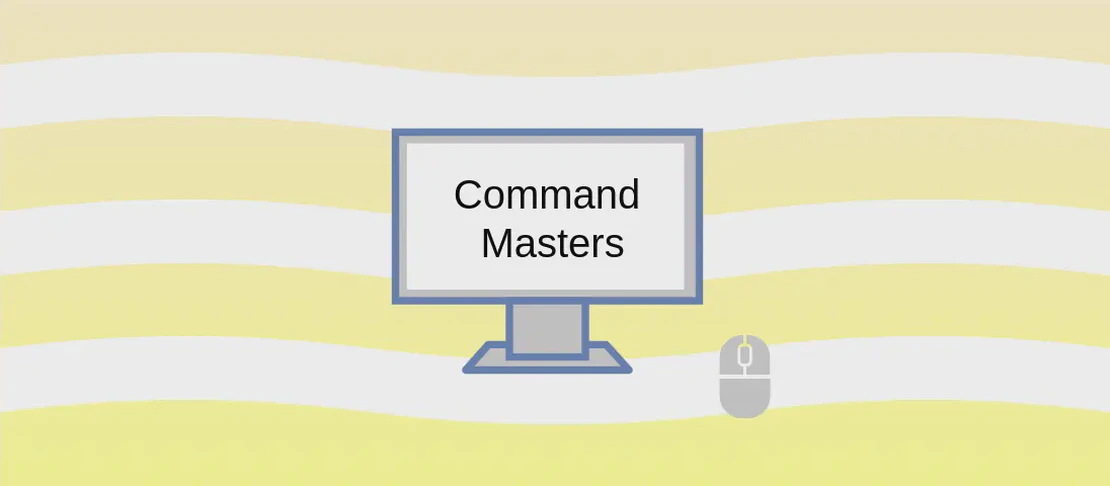
How to use the command Where-Object (with examples)
The Where-Object
cmdlet in PowerShell is a powerful feature that allows you to filter items from a collection based on specified criteria. Users leverage this command to select objects that match certain conditions, thereby streamlining the data management process in scripts or in interactive shell usage. Through Where-Object
, users can specify various conditions related to object properties to extract only relevant data, making operations more efficient and focused.
Use case 1: Filter aliases by its name
Code:
Get-Alias | Where-Object -Property Name -eq name
Motivation:
This use case is focused on filtering PowerShell aliases by their name property. Aliases in PowerShell serve as shorthand representations of commands, and filtering them can aid in organizing and managing how commands are utilized in scripts. It allows users to verify if a specific alias exists or manage aliases more efficiently, ensuring that naming conventions remain consistent and prevent naming conflicts.
Explanation:
Get-Alias
: This command retrieves a list of all aliases currently available in the PowerShell session. Each alias represents a shorter or more user-friendly form of a more extensive command.Where-Object
: With this cmdlet, filtering of the aliases occurs. It sieves through each alias object passed fromGet-Alias
.-Property Name
: Signifies that the filter will be based on the ‘Name’ property of each alias object.-eq name
: Specifies that the objects should be selected where the ‘Name’ property exactly matches ’name’. The-eq
operator stands for ’equals.’
Example Output:
The sample command will return an empty result unless there exists an alias with the exact name “name”. If such an alias exists, the output might look like this:
CommandType Name Definition
----------- ---- ----------
Alias name Some-Command
Use case 2: List all services that are currently stopped
Code:
Get-Service | Where-Object {$_.Status -eq "Stopped"}
Motivation:
There are numerous services running on any Windows-operated machine, and system administrators often need to identify services that have unexpectedly stopped or need maintenance. By listing all stopped services, administrators can quickly diagnose issues, optimize service management, and ensure that necessary services are running as intended, thereby maintaining system reliability.
Explanation:
Get-Service
: This cmdlet fetches a list of services on the system and their current states. It provides a comprehensive overview of all services alongside their properties.Where-Object
: This piece is key for filtering services based on specific criteria.{$_}
: This uses the current object represented by ‘$_’ which stands for each service object passed down the pipeline..Status
: Refers to the ‘Status’ property of each service. Possible values include “Running”, “Stopped”, “Paused”, etc.-eq "Stopped"
: Filters out the services that have their ‘Status’ equal to “Stopped”.
Example Output:
The output will be a list of services with their current status clearly marked as “Stopped”:
Status Name DisplayName
------ ---- -----------
Stopped AppXSvc AppX Deployment Service
Stopped cdpsvc Connected Devices Platform Service
Stopped DiagTrack Connected User Experiences and Telemetry
Use case 3: Use multiple conditions
Code:
Get-Module -ListAvailable | Where-Object { $_.Name -NotLike "Microsoft*" -And $_.Name -NotLike "PS*" }
Motivation:
Modules in PowerShell encompass a range of functionalities, and administrators might want to specifically filter modules that are not part of default distributions, such as those starting with “Microsoft” or “PS”. This could be vital for ensuring that third-party or custom developed modules are working correctly, focusing on user-defined or external modules rather than built-in ones, thus enhancing management and troubleshooting.
Explanation:
Get-Module -ListAvailable
: Generates a list of all modules currently installed on the system. The-ListAvailable
flag ensures you retrieve every module, regardless of whether it is currently loaded.Where-Object
: Filters this comprehensive list based on specified conditions.{ $_.Name -NotLike "Microsoft*"
: Filters out any module whose name starts with “Microsoft”. The-NotLike
operator is used for pattern matching, negating any name that fits the pattern.-And $_.Name -NotLike "PS*"
: A continuation of the filtering process, this condition will also exclude modules whose names start with “PS”, enabling administrators to bring focus on non-default modules.
Example Output:
This command would yield a list of modules excluding those prefixed with “Microsoft” or “PS”, offering clarity on custom or additional modules installed:
ModuleType Version Name ExportedCommands
---------- ------- ---- ----------------
Script 1.0 CustomModule {CustomCommand1, CustomCommand2}
Binary 3.1.0 ThirdPartyTool {ToolCommand}
Conclusion:
The Where-Object
cmdlet is an essential tool in PowerShell for data manipulation and retrieval, offering a method to flexibly and powerfully filter objects based on property values. Whether you are managing aliases, monitoring services, or inspecting available modules, Where-Object
provides the flexibility needed to hone in on specific data, improving efficiency and enabling better system management. The examples and explanations provided illustrate the versatility and usefulness of this cmdlet across various practical use cases.