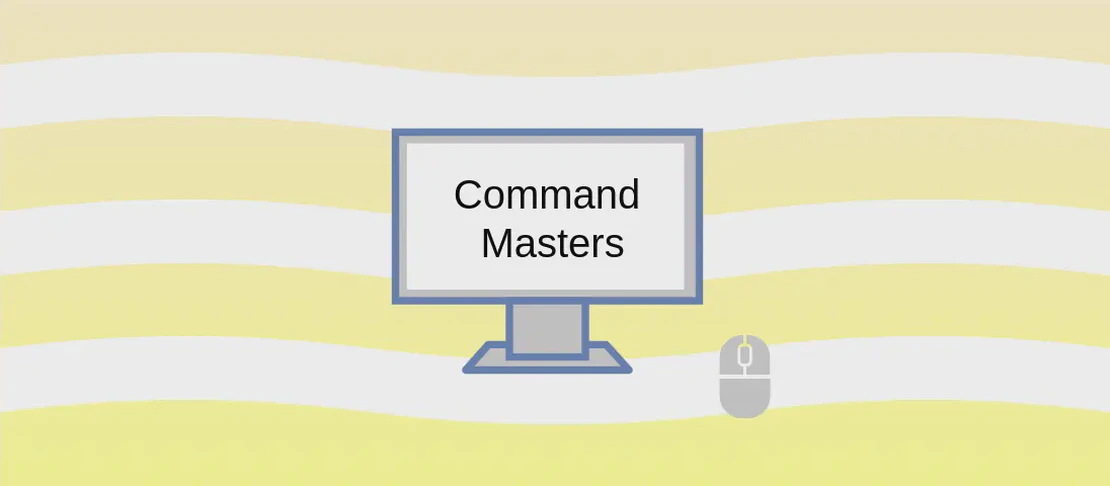
How to Use the Command `while` in Shell Scripting (with Examples)
The while
command is a fundamental construct in shell scripting, used to execute a set of commands repeatedly based on a given condition. It provides a simple loop mechanism where the specified commands run continuously as long as the provided condition evaluates to true. This makes it highly useful for automating repetitive tasks, monitoring system parameters, or processing large datasets.
Use case 1: Read stdin
and Perform an Action on Every Line
Code:
while read line; do echo "$line"; done
Motivation:
This use case is essential when dealing with input streams, such as reading data from a file or interactive user input. By reading each line of input and performing an action, such as printing or transforming the data, we can efficiently handle large datasets or input without the need to store all of it in memory, which is critical in system administration and data processing tasks.
Explanation:
while
: Initiates the loop that will continue executing as long as the specified condition is true.read line
: Reads a single line of input from standard input (stdin) into the variable namedline
.read
is a built-in command that allows for reading a line of text.do
: Begins the block of commands to be executed for each iteration of the loop.echo "$line"
: The action performed on each line, which is simply to print the line to standard output.$line
denotes the content of the variableline
.done
: Marks the end of thewhile
loop.
Example Output:
hello
world
this is a test
If the input provided was:
hello
world
this is a test
The output would echo each line to the console exactly as input.
Use case 2: Execute a Command Forever Once Every Second
Code:
while :; do command; sleep 1; done
Motivation:
There are scenarios in system monitoring or real-time application development where you need to repeatedly execute a command at regular intervals without termination. This kind of loop is vital for tasks such as continuously checking system logs, monitoring server responses, or updating dynamic information like a clock.
Explanation:
while :
: The:
is a built-in command that always returns true, effectively making the loop infinite until manually stopped.do
: Signals the start of the commands to be repeated.command
: Placeholder for any command or script that you wish to execute repeatedly.sleep 1
: Pauses the execution for 1 second before the next iteration starts, ensuring the command runs every second.done
: Concludes thewhile
loop.
Example Output:
Executing command...
Executing command...
Executing command...
This loop will continuously output “Executing command…” every second until it is interrupted.
Use case 3: Execute a Command Until it Returns a Non-zero Value
Code:
while command; do :; done
Motivation:
This structure is particularly useful in scenarios where you want to keep executing a command until a certain error or condition is met, typically used in waiting for a task to complete successfully or retries. For example, you may want to retry a network request until it fails or a system service stops responding.
Explanation:
while
: Begins the loop with the provided condition.command
: Represents any command whose success or failure determines the continuation of the loop. In Unix-like systems, a return value of 0 generally indicates success, while any non-zero value indicates failure.do :
: Using:
as a noop (no operation) means eating up the successful command execution without any action, simply looping again.done
: Ends thewhile
loop.
Example Output:
...
Imagine running a command that pings a server till it fails; nothing will output until the server becomes unreachable, causing the loop to break.
Conclusion:
The while
command is a versatile tool in a shell script’s arsenal, enabling the automation of ongoing and conditional tasks. Its straightforward syntax provides the ability to repeatedly perform actions based on both input data and command success, making it indispensable in controlling flow and logic in scripts, as demonstrated in the examples.