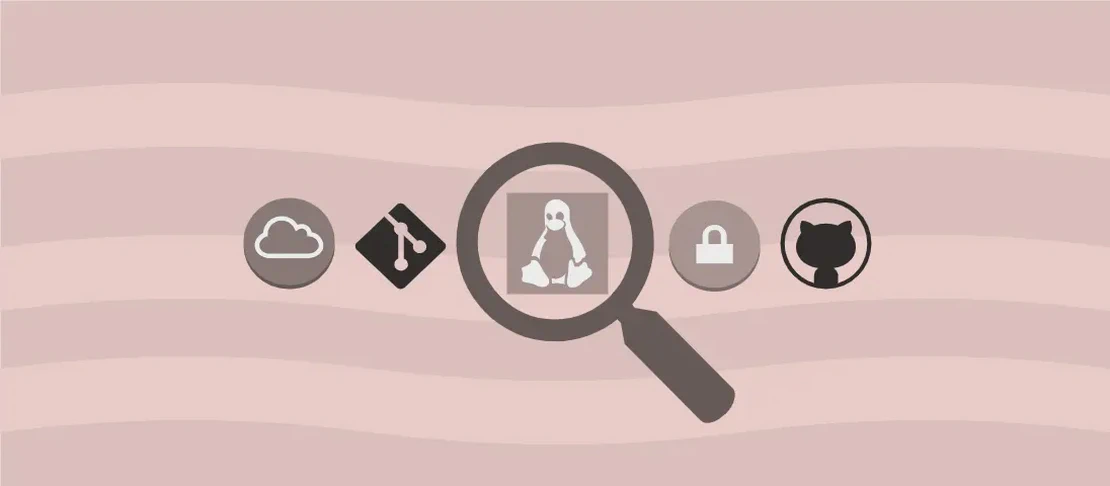
Using the `whiptail` Command (with examples)
- Linux
- December 17, 2024
whiptail
is a command-line utility that allows developers to display text-based dialog boxes in shell scripts. These dialog boxes provide a simple and interactive way to get user input or show messages during script execution. By using whiptail
, scripts can engage users more seamlessly without needing a graphical user interface.
Use case 1: Display a Simple Message
Code:
whiptail --title "Greeting" --msgbox "Hello, World!" 10 30
Motivation:
Imagine you are writing a shell script that automates a lengthy setup process. At the end of the setup, you want to display a confirmation or success message to the user to indicate completion. Displaying a simple message not only confirms the script’s actions but also reassures the user.
Explanation:
--title "Greeting"
: Sets the title of the message box to “Greeting”.--msgbox "Hello, World!"
: Specifies the type of dialog box as a message box and sets the text to “Hello, World!”.10
: Sets the height of the dialog box to 10 lines.30
: Sets the width of the dialog box to 30 characters.
Example Output:
A dialog box with the title “Greeting” will appear on the terminal, displaying the text “Hello, World!” with an OK button to dismiss.
Use case 2: Display a Boolean Choice
Code:
whiptail --title "Confirm" --yesno "Do you want to continue?" 10 30
Motivation:
When a script reaches a point where the user needs to make a decision, such as proceeding with an operation that might overwrite files or making irreversible changes, a yes or no question is appropriate. It allows users to confirm their intentions before proceeding.
Explanation:
--title "Confirm"
: Sets the title of the dialog box to “Confirm”.--yesno "Do you want to continue?"
: Specifies a yes/no dialog box, asking the user if they want to continue.10
: Sets the height of the dialog box to 10 lines.30
: Sets the width of the dialog box to 30 characters.
Example Output:
A dialog box titled “Confirm” will appear, asking “Do you want to continue?” with Yes and No buttons. The script will use the exit code to determine the user’s choice.
Use case 3: Customize Yes/No Button Text
Code:
whiptail --title "Question" --yes-button "Agree" --no-button "Disagree" --yesno "Do you accept the terms?" 10 40
Motivation:
Sometimes, the default Yes/No buttons may not align with the context of the question asked. Customizing the button text to “Agree” and “Disagree” makes the dialog more relevant and understandable to the user.
Explanation:
--title "Question"
: Sets the title of the dialog box to “Question”.--yes-button "Agree"
: Customizes the text of the Yes button to “Agree”.--no-button "Disagree"
: Customizes the text of the No button to “Disagree”.--yesno "Do you accept the terms?"
: Specifies the message in the yes/no dialog box.10
: Sets the height to 10 lines.40
: Sets the width to 40 characters.
Example Output:
A dialog box will ask “Do you accept the terms?” with “Agree” and “Disagree” buttons for user input.
Use case 4: Display a Text Input Box
Code:
user_input="$(whiptail --title "Input" --inputbox "Please enter your name:" 10 30 3>&1 1>&2 2>&3)"
Motivation:
Scripts often need specific input from the user, such as a username or file path. Providing an input box lets users enter text directly, which the script can then use to customize actions or outputs.
Explanation:
--title "Input"
: Sets the dialog box title to “Input”.--inputbox "Please enter your name:"
: Specifies an input box dialog, prompting the user to enter their name.10
: Sets the height to 10 lines.30
: Sets the width to 30 characters.3>&1 1>&2 2>&3
: This line swaps file descriptors to capture the user’s input.
Example Output:
A text input box will appear prompting the user with “Please enter your name:”. The user’s input will be stored in the user_input
variable.
Use case 5: Display a Password Input Box
Code:
user_password="$(whiptail --title "Password" --passwordbox "Enter your password:" 10 30 3>&1 1>&2 2>&3)"
Motivation:
When scripts require sensitive information such as passwords, using a password input box ensures that the input isn’t displayed on the screen, thus maintaining user privacy.
Explanation:
--title "Password"
: Sets the title of the dialog box to “Password”.--passwordbox "Enter your password:"
: Specifies a password input box dialog.10
: Sets the height to 10 lines.30
: Sets the width to 30 characters.3>&1 1>&2 2>&3
: Redirects output to get the input securely.
Example Output:
A password input box will be displayed asking “Enter your password:”. The input will be stored in the user_password
variable without showing any text as it’s typed.
Use case 6: Display a Multiple-Choice Menu
Code:
choice=$(whiptail --title "Choose Option" --menu "Select an option:" 15 50 4 "1" "Option 1" "2" "Option 2" "3" "Option 3" "4" "Option 4" 3>&1 1>&2 2>&3)
Motivation:
For scripts that offer multiple options to choose from, a menu provides a convenient and organized way to present these choices. This use case is particularly useful when configuring applications or selecting settings.
Explanation:
--title "Choose Option"
: Sets the dialog box title to “Choose Option”.--menu "Select an option:"
: Specifies a menu-style dialog box with the message “Select an option:”.15
: Sets the height to 15 lines.50
: Sets the width to 50 characters.4
: Specifies the display height for the menu to show 4 options at a time."1" "Option 1" "2" "Option 2" "3" "Option 3" "4" "Option 4"
: Defines the list of options presented in the menu.3>&1 1>&2 2>&3
: Redirects output to capture the user’s choice.
Example Output:
A menu will be presented with options labeled “Option 1”, “Option 2”, “Option 3”, and “Option 4”. The user’s selection is stored in the choice
variable.
Conclusion:
whiptail
provides a versatile way to add interactive elements to shell scripts, enhancing user experience while maintaining simplicity. These dialog boxes can vary from simple messages to complex menus, allowing scripts to handle user input in a more controlled and user-friendly manner. Whether prompting for confirmation or gathering sensitive information, whiptail
ensures your scripts are both functional and engaging.