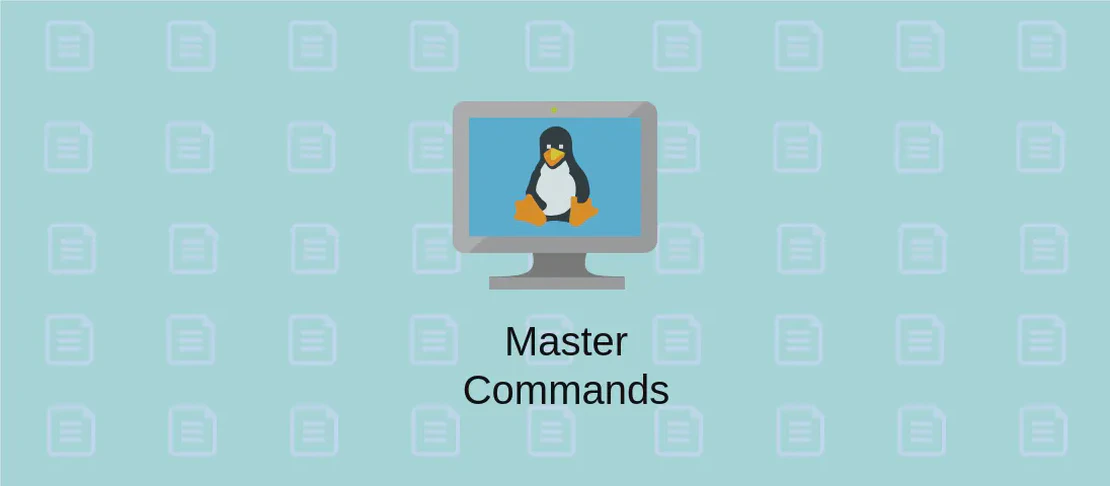
Navigating the Command 'wsl' on Windows Subsystem for Linux (with examples)
- Windows
- December 17, 2024
The Windows Subsystem for Linux (WSL) provides a robust environment for running a GNU/Linux distribution directly on Windows—without requiring a dual boot. Closing the gap between Linux and Windows, WSL integrates Linux commands and scripts seamlessly into the Windows environment. Below, we will explore various use cases of the ‘wsl’ command, each with detailed explanations and example outputs to help you become proficient in leveraging WSL to its full potential.
Start a Linux shell (in the default distribution)
Code:
wsl
Motivation:
Starting a Linux shell using WSL provides users the familiar Linux command line interface where they can perform various tasks including file manipulation, running scripts, or exploring Linux-based projects directly from Windows. This is particularly useful for developers who want to utilize powerful Linux tools and utilities from within their Windows environment.
Explanation:
By simply typing wsl
, you open the default Linux distribution installed on your system. Without any arguments, the command defaults to opening a shell instance, giving you access to the Linux environment.
Example Output:
Upon executing the command, the shell prompts you within the Linux environment:
user@host:~$
This prompt indicates you’ve successfully entered the Linux shell in your default distribution.
Run a Linux command without using a shell
Code:
wsl --exec ls /
Motivation:
Running a command directly without opening a full shell is an efficient way to execute single tasks or scripts. This save time and system resources by running only the specified command and then immediately exiting.
Explanation:
The --exec
option allows users to pass a specific command to be executed. In this example, ls /
lists the contents of the root directory. This instance of executing commands is powerful for automation scripts, allowing for seamless integration of Linux commands in various workflows.
Example Output:
The command returns the directory listing of the root filesystem:
bin boot dev etc home lib lib64 media mnt opt proc root run sbin srv sys tmp usr var
Specify a particular distribution
Code:
wsl --distribution Ubuntu ls /home
Motivation:
For users who manage multiple Linux distributions, specifying the exact one to use is essential. This capability allows a targeted approach, enabling usage of different distributions depending on development needs or software compatibilities.
Explanation:
The --distribution
parameter specifies the Linux distribution to target. In this case, Ubuntu
is chosen. The command ls /home
lists the contents of the home directory within this specified distribution, demonstrating usage beyond the default environment.
Example Output:
Contents of the /home
directory in the Ubuntu distribution:
user1 user2 shared
List available distributions
Code:
wsl --list
Motivation:
Listing distributions helps users keep track of the multiple environments installed on their system. It showcases the available choices at your disposal for when you need to work with a specific Linux distribution.
Explanation:
The --list
parameter displays all the Linux distributions that are currently installed. This is crucial for maintaining transparency and providing management capabilities over your WSL instances.
Example Output:
A list of distributions installed on your system:
Ubuntu-20.04 (Default)
Debian
Kali-linux
Export a distribution to a .tar
file
Code:
wsl --export Ubuntu-20.04 C:\Users\user\Desktop\ubuntu_backup.tar
Motivation:
Exporting a distribution to a .tar
file is crucial for creating backups or moving distributions between machines. This ensures data and environment settings are preserved and can be replicated or restored easily.
Explanation:
The --export
command takes two arguments: the name of the distribution Ubuntu-20.04
and the path to save the .tar
file C:\Users\user\Desktop\ubuntu_backup.tar
. This creates a portable archive of your Linux environment.
Example Output:
There is no output, but you will find ubuntu_backup.tar
at the specified path, preserving your distribution for future use.
Import a distribution from a .tar
file
Code:
wsl --import Ubuntu-Clone C:\WSL\Ubuntu-Clone C:\Users\user\Desktop\ubuntu_backup.tar
Motivation:
Importing a distribution allows users to recreate or share their environments easily. This is useful for developers who need consistent environments across different machines or who wish to distribute a pre-configured Linux environment.
Explanation:
The --import
option takes three arguments. The name Ubuntu-Clone
is how the distribution will appear in your list, the location C:\WSL\Ubuntu-Clone
where the distribution will be installed, and the .tar
file C:\Users\user\Desktop\ubuntu_backup.tar
from which to import.
Example Output:
Again, there is no output, but the distribution Ubuntu-Clone
is now available and ready for use.
Change the version of WSL used for the specified distribution
Code:
wsl --set-version Ubuntu-20.04 2
Motivation:
Changing the WSL version can optimize performance or enhance compatibility with software. WSL 2 offers a full Linux kernel, providing greater performance and full system call compatibility, which is essential for more complex development tasks.
Explanation:
The --set-version
flag includes two arguments: the distribution Ubuntu-20.04
and the desired WSL version 2
. This switches the chosen distribution from WSL 1 to WSL 2, providing enhanced features and performance capabilities.
Example Output:
There is no direct output, but running the version command again will confirm that the distribution version has switched appropriately.
Shut down Windows Subsystem for Linux
Code:
wsl --shutdown
Motivation:
Shutting down WSL is sometimes necessary to free up system resources or resolve issues like stuck processes. Like restarting a system, shutting down all WSL distributions can be a convenient quick-fix.
Explanation:
The --shutdown
command stops all running WSL instances. It’s a global command that applies across all distributions, acting as a reset of sorts for the WSL ecosystem on your machine.
Example Output: This command runs silently without output but ensures that all WSL processes are terminated.
Conclusion:
These illustrative examples of the wsl
command in various scenarios reveal the flexibility and power of WSL within a Windows environment. By utilizing these commands, users can create, manage, and deploy Linux environments seamlessly alongside their existing Windows systems. Whether for development, testing, or personal projects, WSL provides an essential bridge between these two worlds, simplifying workflows and broadening possibilities.