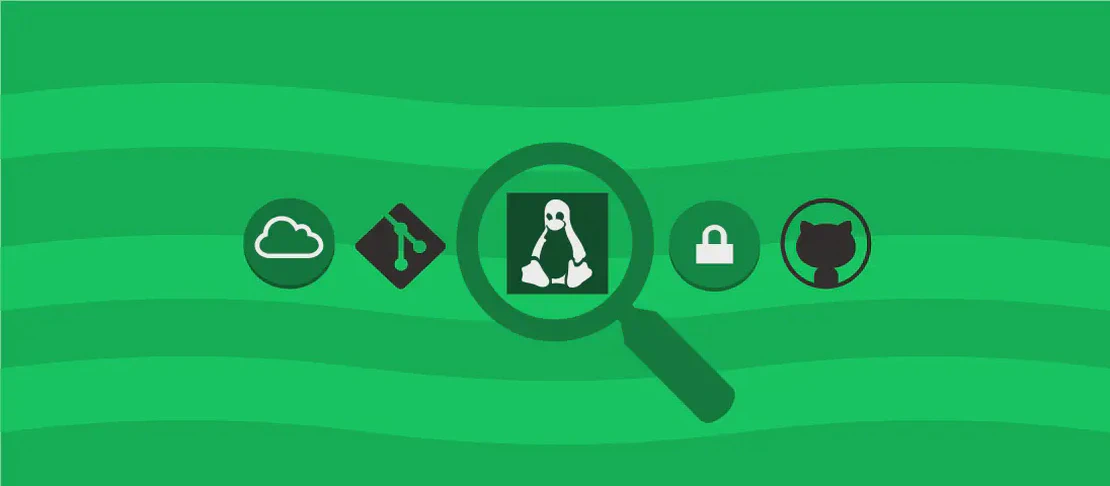
How to use the command 'xcodebuild' (with examples)
- Osx
- December 17, 2024
The xcodebuild
command is a powerful tool that allows developers to build Xcode projects from the command line. This command is particularly useful for automating build processes, integrating with continuous integration (CI) systems, and managing several build configurations and schemes. It provides a flexible way to programmatically handle various aspects of Xcode build workflows. Below, we will explore different use cases of the xcodebuild
command, offering step-by-step explanations and examples.
Build Xcode Workspace: Building a Workspace
Code:
xcodebuild -workspace workspace_name.workspace -scheme scheme_name -configuration configuration_name clean build SYMROOT=SYMROOT_path
Motivation: Building an Xcode workspace using the command line is often essential for large projects that are divided into multiple components. By using a command like this, developers can automate the build process or integrate it into a CI/CD pipeline. This ensures consistency across builds and reduces the chance of human error that can occur in manual build processes.
Explanation:
-workspace workspace_name.workspace
: This option specifies the path to the Xcode workspace file (.xcworkspace) that you want to build. Workspaces are ideal for organizing multiple related projects, allowing for modular development.-scheme scheme_name
: The scheme specifies a set of instructions for building a product, including its configuration, build targets, and testing setup. It ensures that the intended configuration is used during the build process.-configuration configuration_name
: Defines the build configuration to use, such as Debug, Release, etc. Different configurations are used for development, testing, and final production builds.clean build
: This sequence of arguments tellsxcodebuild
to first clean the build environment (removing any previous build artifacts) and then proceed to build the workspace. This ensures that no remnants of previous builds interfere with the current build.SYMROOT=SYMROOT_path
: Specifies the directory where build products and intermediate files should be placed. This is useful for organizing outputs, particularly in a CI setup where builds occur in temporary environments.
Example Output:
=== BUILD TARGET YourAppName OF PROJECT YourProjectName WITH CONFIGURATION Debug ===
Check dependencies
...
** BUILD SUCCEEDED **
Build Xcode Project: Building a Project
Code:
xcodebuild -target target_name -configuration configuration_name clean build SYMROOT=SYMROOT_path
Motivation: When working with individual projects rather than workspaces, a developer can use this specific command to handle builds. This is common in smaller projects or when focusing on a single component of a larger system. By leveraging command-line builds, developers can run automated tests and deploy applications seamlessly as part of a continuous integration process.
Explanation:
-target target_name
: This defines the specific target to build within the Xcode project. Targets represent a single end product in a project, and choosing the appropriate one ensures that the build process conforms to that product’s requirements.-configuration configuration_name
: Similar to the workspace build, this specifies which configuration (such as Debug or Release) the build process should use.clean build
: Instructsxcodebuild
to first clean existing build artifacts before initiating a new build. Cleaning the build helps resolve issues related to old cached files affecting new builds.SYMROOT=SYMROOT_path
: Directs where to output build files and products. Setting a specific directory aids in organizing build outputs and ensures a cleaner filesystem, particularly in automated environments.
Example Output:
=== BUILD TARGET YourTargetName OF PROJECT YourProjectName WITH CONFIGURATION Release ===
Check dependencies
...
** BUILD SUCCEEDED **
Show Available SDKs: Listing SDKs
Code:
xcodebuild -showsdks
Motivation: Revealing the list of installed SDKs provides developers with critical information about the tools available to build their projects. This can be crucial when targeting specific iOS versions or features that require particular SDKs. Knowing available SDKs helps ensure compatibility and performance optimization during app development.
Explanation:
-showsdks
: This flag tellsxcodebuild
to list all the installed Software Development Kits (SDKs) available for building projects. It’s particularly useful for developers who need to confirm which platforms and versions can be targeted from their system.
Example Output:
iOS SDKs:
iOS 15.0 -sdk iphoneos15.0
iOS Simulator SDKs:
Simulator - iOS 15.0 -sdk iphonesimulator15.0
macOS SDKs:
macOS 12.0 -sdk macosx12.0
tvOS SDKs:
tvOS 15.0 -sdk appletvos15.0
tvOS Simulator SDKs:
Simulator - tvOS 15.0 -sdk appletvsimulator15.0
watchOS SDKs:
watchOS 8.0 -sdk watchos8.0
watchOS Simulator SDKs:
Simulator - watchOS 8.0 -sdk watchsimulator8.0
Conclusion:
The xcodebuild
command is an invaluable tool for developers working with Xcode projects, providing a command-line interface to manage and execute builds. By understanding and utilizing the examples given above, developers can improve their development workflows, ensure robust build processes, and maintain control over their project environments. Whether for integrating into CI pipelines or managing build configurations effectively, xcodebuild
unlocks significant flexibility and efficiency in software development.