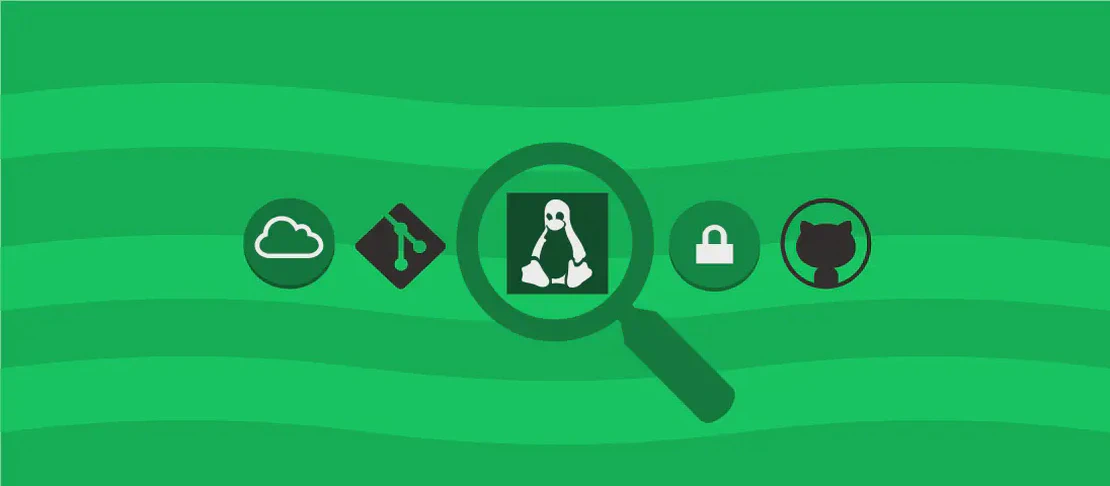
How to Use the Command 'xmlstarlet' (with Examples)
Xmlstarlet is a versatile command-line XML/XSLT toolkit, designed to improve the way users work with XML documents. It allows users to read, edit, manipulate, and create XML content efficiently from the terminal. It’s essential for those working extensively with XML files in scripts or on the command line.
Xmlstarlet is particularly powerful because it leverages the XPath language, which is pivotal for XML navigation and manipulation. Users can thereby harness the power of XPath expressions to target and work with specific parts of their XML data.
Use Case 1: Format an XML Document and Print to stdout
Code:
xmlstarlet format path/to/file.xml
Motivation:
Formatting XML documents can significantly enhance their readability and organization, especially when dealing with unformatted or minified files. This is crucial for developers or analysts who need to quickly interpret and understand the structure and content of XML data.
Explanation:
xmlstarlet
: Invokes the Xmlstarlet command-line toolkit.format
: Specifies the action of formatting the XML document for better readability.path/to/file.xml
: This is the path to the XML file that you wish to format.
Example Output:
A minified XML might look like:
<root><element key="value">text</element><element>text2</element></root>
After formatting, it might look like:
<root>
<element key="value">text</element>
<element>text2</element>
</root>
Use Case 2: XML Document Can Also Be Piped from stdin
Code:
cat path/to/file.xml | xmlstarlet format
Motivation:
Using piping to format XML documents is highly beneficial when integrating Xmlstarlet with other command-line utilities. This allows a streamlined and efficient workflow in command-line environments, providing the ability to pass XML data through various transformations in a single chained command.
Explanation:
cat path/to/file.xml
: Outputs the contents of the specified XML file.|
: Pipes the output ofcat
into the next command.xmlstarlet format
: Formats the piped XML input.
Example Output:
Similar formatted output as in Use Case 1 since the XML content is being piped in instead.
Use Case 3: Print All Nodes that Match a Given XPath
Code:
xmlstarlet select --template --copy-of xpath path/to/file.xml
Motivation:
This use case is particularly useful for extracting specific nodes from a larger XML document based on an XPath query. It’s valuable for scenarios where only a subset of data is needed without manually sifting through the XML.
Explanation:
select
: The subcommand for selecting parts of the XML document.--template --copy-of xpath
: Outputs the matched nodes as defined by the XPath expression.path/to/file.xml
: Specifies the XML file to be parsed.
Example Output:
If the XML document has multiple <item>
nodes and you use the XPath //item
, the output will be all the <item>
nodes.
Use Case 4: Insert an Attribute to All Matching Nodes, and Print to stdout
(Source File is Unchanged)
Code:
xmlstarlet edit --insert xpath --type attr --name attribute_name --value attribute_value path/to/file.xml
Motivation:
There may be circumstances where one needs to add attributes dynamically to XML nodes based on specific conditions or queries. This helps adapt XML data for various use cases without altering the original document, preserving data integrity.
Explanation:
edit
: Indicates an edit action without changing the source.--insert xpath
: Specifies the path where the attribute will be inserted.--type attr
: Denotes that the type of insertion is an attribute.--name attribute_name --value attribute_value
: Details the attribute name and value to be inserted.path/to/file.xml
: The XML file upon which the operation is conducted.
Example Output:
Original:
<element>text</element>
After command execution:
<element attribute_name="attribute_value">text</element>
Use Case 5: Update the Value of All Matching Nodes In Place (Source File is Changed)
Code:
xmlstarlet edit --inplace --update xpath --value new_value file.xml
Motivation:
When consistent changes need to be applied across an XML document, this command does just that. It’s crucial for keeping data updated and relevant without needing manual intervention across multiple nodes or documents.
Explanation:
edit
: Invokes edit mode with changes directly in the file.--inplace
: Applies changes directly to the specified file.--update xpath --value new_value
: Updates nodes found by the XPath with the new value.file.xml
: The document to modify.
Example Output:
If /root/node
contains “old value”, it will be replaced with “new value”.
<root>
<node>new value</node>
</root>
Use Case 6: Delete All Matching Nodes In Place (Source File is Changed)
Code:
xmlstarlet edit --inplace --delete xpath file.xml
Motivation:
Deleting nodes based on a query can clean up XML documents from unwanted data efficiently. It’s essential for managing and maintaining XML data integrity over time, removing obsolete or redundant entries.
Explanation:
edit
: Enters edit mode to modify the file.--inplace
: Specifies that changes should be saved to the original document.--delete xpath
: Deletes nodes matched by the XPath.file.xml
: The file you wish to modify.
Example Output:
If nodes matched by the XPath are removed, such as <node>
, they won’t appear in the revised XML document.
Use Case 7: Escape or Unescape Special XML Characters in a Given String
Code:
xmlstarlet escape 'some <encoded> string'
Motivation:
Handling special characters correctly is pivotal in XML processing. This use case supports converting special characters to XML-safe sequences and vice versa, thus ensuring the XML content remains valid and correctly interpretable by parsers.
Explanation:
escape
: Converts special characters into their equivalent entity codes.- Alternatively,
unescape
reverts encoded entities back to characters.
Example Output:
When using escape
, the input:
some <encoded> string
Will output:
some <encoded> string
Use Case 8: List a Given Directory as XML (Omit Argument to List Current Directory)
Code:
xmlstarlet ls path/to/directory
Motivation:
Representing filesystem data as XML can facilitate integration with other XML workflows and applications. This is particularly useful for systems that interface directly with directory structures and need to process or visualize them in an XML format.
Explanation:
ls
: Lists the directory contents.path/to/directory
: Specifies the directory whose contents are to be listed in XML format.
Example Output:
The XML output for a directory listing might resemble:
<dir name="path/to/directory">
<file>file1.txt</file>
<file>file2.txt</file>
</dir>
Conclusion:
Xmlstarlet is an invaluable tool for anyone dealing with XML data at the command line. With its capability to format, edit, query, and more, Xmlstarlet allows for sophisticated XML manipulation and transformation, empowering users to maintain and work with XML data efficiently. By understanding and implementing these use cases, users can better manage XML data within various workflows and pipelines.