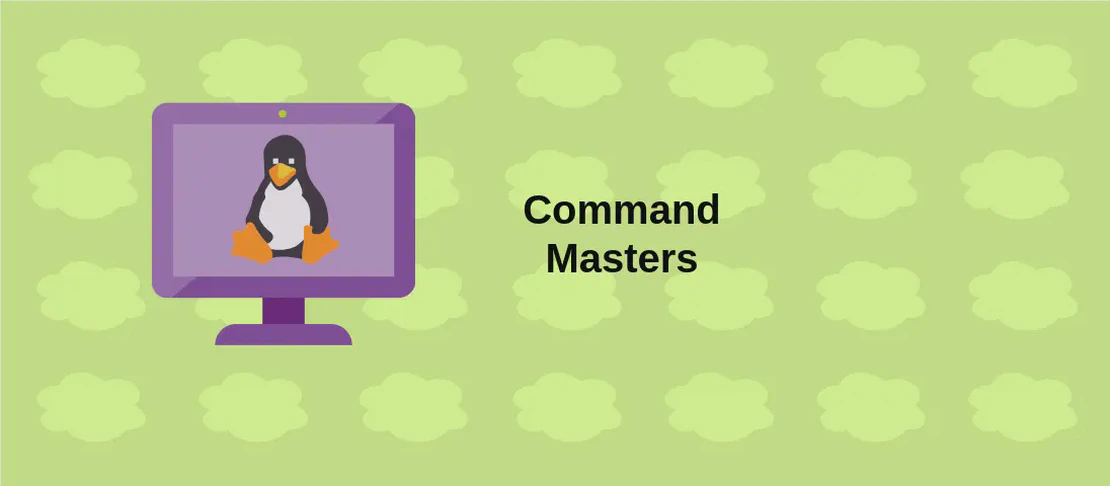
Effective JavaScript Linting with 'xo' (with examples)
“xo” is a powerful linting tool for JavaScript that provides developers with the ability to maintain consistent and error-free code. As a zero-configuration utility, it integrates seamlessly into a project, offering flexibility through its pluggable architecture. With its simplicity and the array of customization options it offers, “xo” is an excellent choice for developers aiming to improve code quality in their projects.
Lint files in the “src” directory
Code:
xo
Motivation:
Linting is an essential practice in software development, particularly for identifying and fixing problematic patterns or styles in your code. By running xo
, you can statically analyze the code in the “src” directory, detecting potential errors, ensuring adherence to coding standards, and improving code maintainability. This step is crucial for any development workflow, providing a baseline check of code quality.
Explanation:
xo
: The commandxo
by itself defaults to lint all the JavaScript files within the current working directory. In this context, it will check the “src” directory for any JavaScript files that need linting attention. This automates the initial review of all files, saving time and reducing manual oversight.
Example Output:
src/index.js:2:1
error Use the global form of 'use strict'. strict
src/modules/utils.js:15:5
warning Expected error to be handled. handle-callback-err
✖ 2 problems (1 error, 1 warning)
Lint a given set of files
Code:
xo path/to/file1.js path/to/file2.js ...
Motivation:
When working on particular files or modules, it is sometimes unnecessary to lint the entire codebase. You might want to focus your attention on specific files individually, especially if they have seen recent changes or are critical parts of the application. This specific command allows developers to pinpoint their linting to chosen files rather than reviewing the entire directory, thus optimizing the development process by concentrating resources where they are needed most.
Explanation:
xo path/to/file1.js path/to/file2.js ...
: By providing a set of file paths to thexo
command, you direct it to focus only on those files. This approach is particularly practical when modifications are isolated or if you’re debugging a specific issue within these files.
Example Output:
path/to/file1.js:8:10
error 'console' is not allowed. no-console
path/to/file2.js:20:23
warning Strings must use single quotes. quotes
✖ 2 problems (1 error, 1 warning)
Automatically fix any lint issues found
Code:
xo --fix
Motivation:
Handling codebases manually for lint issues can be tedious. The --fix
option provides a significant improvement in efficiency by automating the resolution of many common issues. This is particularly useful in large-scale projects where minor violations are abundant, ensuring that routine problems are corrected rapidly without human intervention while adhering to the defined coding standards.
Explanation:
xo --fix
: The--fix
argument instructsxo
to attempt automatic fixes on any lint issues it encounters. This often includes formatting corrections such as spacing, quote usage, and minor syntax adjustments that do not require human attention. It simplifies the developer’s workload by reducing the number of issues they need to fix manually.
Example Output:
success Automatically fixed 5 problems
Lint using spaces as indentation instead of tabs
Code:
xo --space
Motivation:
Code styling preferences vary across different teams and projects. While some prefer tabs, others opt for spaces to indent their code. Consistency in this styling is crucial for readability and maintenance, particularly in collaborative environments. The --space
option enforces spaces over tabs, ensuring that contributors who are accustomed to using spaces can easily align the code to their preferences.
Explanation:
xo --space
: This option tellsxo
to enforce the use of spaces instead of tabs for indentation in your code. It helps maintain a uniform coding style throughout the project, which is especially vital when onboarding new team members who may have different preferences.
Example Output:
3 problems (2 errors, 1 warning)
2 errors and 0 warnings potentially fixable with the `--fix` option
Lint using the “prettier” code style
Code:
xo --prettier
Motivation:
“Prettier” is a highly popular code formatter that enforces a consistent style by parsing your code and re-printing it with its own rules. By integrating “Prettier” with xo
, developers can ensure that their code meets the widespread, standard presets, which adds an extra layer of appeal due to its widespread acceptance and usage in multiple coding communities. This option contributes immensely towards unifying code style, regardless of personal coding preferences.
Explanation:
xo --prettier
: This option modifies thexo
configuration to employ “Prettier” as its style guide. By doing so, it aligns the project’s codebase with the stylistic choices made by “Prettier,” promoting clean and consistent formatting, while abstracting style concerns away from developers.
Example Output:
Checked 26 files.
No code style issues found.
Conclusion:
The “xo” command offers a robust and versatile linting solution for JavaScript developers, enhancing productivity and code quality significantly. Through its various options, xo
can adapt to different coding and stylistic needs, from specific issues resolution with the --fix
command to comprehensive code style enforcement with “Prettier.” Regular usage of these commands ensures that code remains clean, error-free, and maintainable across the lifespan of a project.