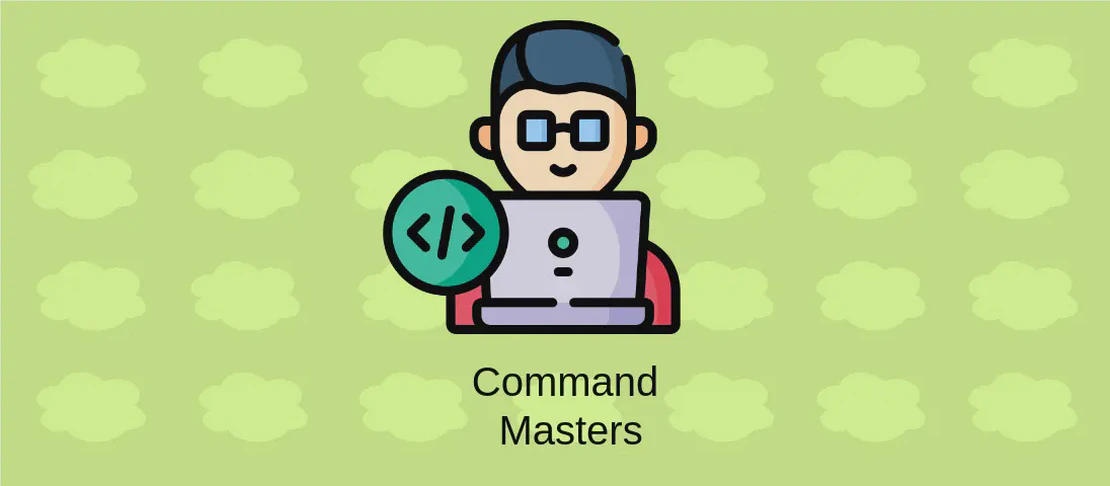
How to use the command 'yapf' (with examples)
YAPF, or Yet Another Python Formatter, is a powerful tool developed by Google to automatically format Python code according to specified style guide rules. It can help maintain consistency and readability across a codebase by ensuring that all contributed code adheres to a predetermined style, reducing the cognitive load when switching between projects or collaborating with multiple contributors. YAPF can be tailored to comply with different style guides, such as PEP 8, or customized through configuration files.
Use case 1: Displaying a diff of proposed changes without modifying the file
Code:
yapf --diff path/to/file
Motivation:
There are times when developers want to see what changes YAPF would make to their code without actually altering the file. This is often the case during initial evaluations of code formatting solutions or when new team members are getting acquainted with YAPF’s behavior. By previewing the potential changes, developers can assess whether YAPF meets their needs or whether additional configuration is necessary. This command is an excellent starting point for integrating YAPF into an existing codebase without committing to immediate modifications.
Explanation:
yapf
: Invokes the YAPF formatter.--diff
: This option instructs YAPF to output a unified diff of the changes it would make, rather than applying them directly. A unified diff is a text format that shows line-by-line changes with context around each change.path/to/file
: Specifies the file on which YAPF will perform its analysis, directing it to check a specific file for formatting inconsistencies.
Example Output:
@@ -1,5 +1,5 @@
def example_function(a, b):
- x = { 'key':value }
+ x = {'key': value}
return x
Use case 2: Formatting the file in-place and displaying a diff of the changes
Code:
yapf --diff --in-place path/to/file
Motivation:
When developers are confident about the format changes YAPF enforces, they might choose to apply those changes directly to their files. The --in-place
option accomplishes this by overwriting the original file with the formatted code. Having --diff
partnered with --in-place
serves a dual purpose: it not only updates the code but also displays the modifications made in the terminal, providing real-time insight into what adjustments were necessary. This approach is useful in maintaining transparency and ensuring that automated changes align with the developer’s expectations.
Explanation:
yapf
: Executes the YAPF command.--diff
: Displays the differences between the original and the new file within the terminal.--in-place
: Tells YAPF to directly modify the file, replacing its contents with the formatted version.path/to/file
: Denotes the file path that YAPF should format in place.
Example Output:
@@ -1,5 +1,5 @@
def example_function(a, b):
- x = { 'key':value }
+ x = {'key': value}
return x
Use case 3: Recursively formatting all Python files in a directory, concurrently
Code:
yapf --recursive --in-place --style pep8 --parallel path/to/directory
Motivation:
For larger projects with multiple files and directories, formatting them individually is not practical. This command is essential for efficiently applying YAPF to an entire codebase. It recursively scans through directories and sorts out the Python files that need formatting. By running it in parallel, it leverages multiple CPU cores, thereby speeding up the process. Using this command ensures a uniform style across the entire project directory, optimizing the coding standard in line with PEP 8 effortlessly.
Explanation:
yapf
: Initiates the YAPF formatting tool.--recursive
: Instructs YAPF to recursively find and format all Python files within the specified directory.--in-place
: Directs YAPF to make changes directly to each file.--style pep8
: Specifies the coding style to be applied, which in this case, is the widely-accepted PEP 8 style guide.--parallel
: This enables YAPF to process multiple files concurrently by utilizing available CPU cores, significantly reducing execution time.path/to/directory
: Points to the directory which contains all the Python files YAPF needs to format recursively.
Example Output:
Reformatted path/to/directory/file1.py
Reformatted path/to/directory/file2.py
...
Conclusion:
YAPF is an invaluable tool for Python developers seeking to maintain consistent coding styles across single files or entire projects. Whether the goal is to preview potential changes, make in-place adjustments, or enforce a style recursively on a directory, YAPF provides versatile options. These capabilities ease the code review process, promote readability, and can dramatically reduce the time spent on nitpicking stylistic inconsistencies in Python codebases.