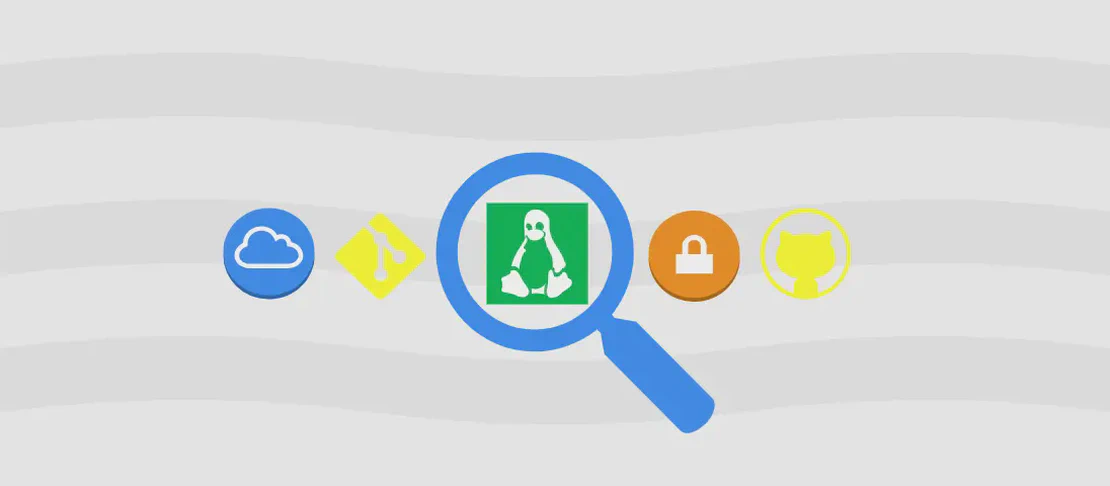
Mastering Yarn: Understanding Its Key Use Cases (with examples)
Yarn is a powerful package manager that provides an alternative to npm for managing JavaScript and Node.js project dependencies. With its focus on speed, stability, and reliability, Yarn helps developers manage their project dependencies with ease and efficiency. This article delves into various use cases of the Yarn command, illustrating the diverse ways it can be employed to manage packages in your Node.js projects.
Install a Module Globally
Code:
yarn global add module_name
Motivation:
Global modules are accessible from any directory on your system, which makes them convenient for running command-line tools and utilities. Installing a module globally is particularly useful when you need a tool available for your entire development environment, like a testing framework or a CLI tool generator, without having to install it in each individual project.
Explanation:
yarn
: The command-line utility.global
: This argument tells Yarn to install the package globally, making it accessible across all projects and sessions.add
: This is the command used to install a module.module_name
: The name of the module you want to install globally. Replacemodule_name
with the actual module’s name.
Example Output:
If you run this command and it completes successfully, you would see a confirmation message indicating that the module has been added globally, such as:
success Installed "module_name" with binaries available locally to the environment.
Install All Dependencies Referenced in the Package.json File
Code:
yarn install
Motivation:
Before running or developing a project, it is essential to ensure that all the required dependencies are installed. When you clone a project from a repository with no node_modules
present, this command is used to download all the packages specified in the package.json
file, ensuring the project has all necessary dependencies configured.
Explanation:
yarn
: The main command-line utility.install
: This command will read from thepackage.json
file and install all of the declared dependencies within it. Theinstall
is optional since simply runningyarn
in a directory with apackage.json
present will default to this behavior.
Example Output:
Running this command typically results in:
yarn install v1.x.x
[1/4] 🔍 Resolving packages...
[2/4] 🚚 Fetching packages...
[3/4] 🔗 Linking dependencies...
[4/4] 🔨 Building fresh packages...
✨ Done in x.xx seconds.
Install a Module and Save it as a Dependency to the Package.json File
Code:
yarn add module_name@version
Motivation:
Adding dependencies is a common practice when developing new features. Installing a specific version of a module as a dependency keeps the environment stable and consistent, aligning with other features and tools within your project.
Explanation:
yarn
: Initiates the command.add
: This denotes that a module is being added as part of the project’s dependency list.module_name
: The module you expect to install.@version
: Specifies the exact version of the package you wish to install. If omitted, Yarn installs the latest version.
Example Output:
yarn add v1.x.x
[1/4] 🔍 Resolving packages...
[2/4] 🚚 Fetching packages...
[3/4] 🔗 Linking dependencies...
[4/4] 🔨 Building fresh packages...
success Saved lockfile.
success Saved "module_name@version" to "dependencies".
✨ Done in x.xx seconds.
Uninstall a Module and Remove it from the Package.json File
Code:
yarn remove module_name
Motivation:
Projects evolve, and certain packages might become redundant, obsolete, or replaced by alternatives. Removing such dependencies helps clean up the codebase and minimize security risks by reducing your project’s attack surface.
Explanation:
yarn
: Launches the command utility.remove
: This command is used for removing a package from the project.module_name
: The specific module you intend to remove from your project.
Example Output:
yarn remove v1.x.x
[1/2] 🗑️ Removing module_name...
[2/2] 🔗 Regenerating lockfile and installing missing dependencies...
success Uninstalled "module_name".
✨ Done in x.xx seconds.
Interactively Create a Package.json File
Code:
yarn init
Motivation:
Starting a new project requires structuring it properly from the outset. The package.json
file is central in defining the project’s requirements, scripts, and dependencies. Creating one interactively helps tailor this file to your specific project needs while documenting them at the starting phase.
Explanation:
yarn
: Invokes the command utility.init
: This launches an interactive questionnaire that helps you configure a newpackage.json
file according to responses provided by the user.
Example Output:
This prompts a series of questions where you specify things like the project name, version, and entry point. At the end, you get a confirmation:
success Saved package.json
Identify Whether a Module is a Dependency and List Other Modules that Depend Upon It
Code:
yarn why module_name
Motivation:
Understanding why a specific package is included in the project and what other packages rely on it is crucial for effective dependency management. This is particularly useful for troubleshooting conflicts or when auditing security vulnerabilities tied to specific modules.
Explanation:
yarn
: Initiates the command.why
: Asks Yarn to explain why a particular module is present in the project.module_name
: The name of the module in question.
Example Output:
Example output may include details such as:
[1/4] Why do we have the module_name...
=> Found "module_name#version"
info Reasons this module exists
- "another_module#version" depends on it
info Disk size with unique dependencies: "some_size"
info Disk size with transitive dependencies: "some_size"
info Number of shared dependencies: "some_number"
Conclusion
Understanding and utilizing Yarn’s capabilities can dramatically increase efficiency and control within your JavaScript and Node.js projects. From installing and removing packages to managing entire project dependencies, Yarn provides clear, intuitive commands to streamline your package management process. This understanding ensures that as a developer, you can maintain high project standards, address dependencies accurately, and stay focused on delivering high-quality code.