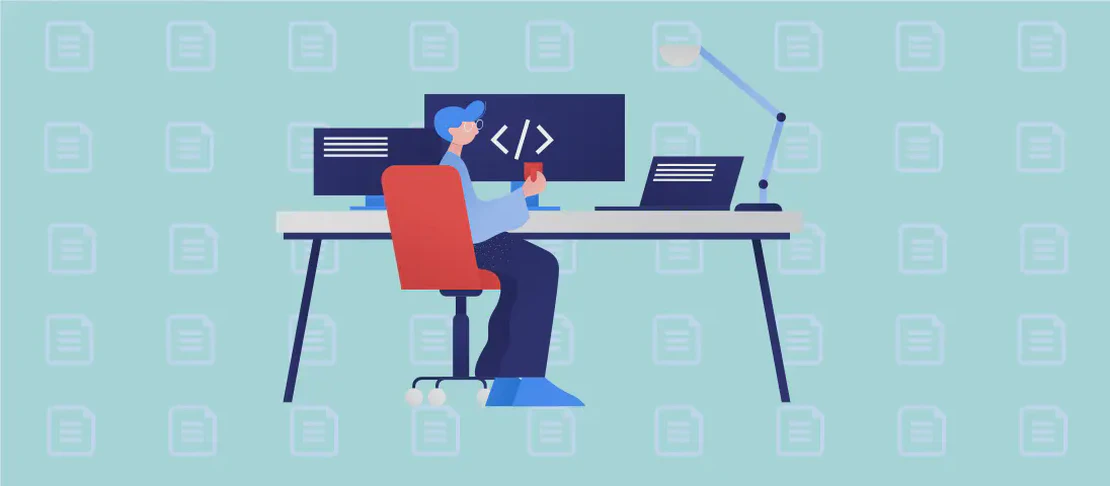
Mastering the Command-Line with 'yq' (with examples)
The yq
command is a powerful, lightweight, and highly portable command-line tool designed to parse, manipulate, and transform YAML files. The simplicity and flexibility offered by yq
cater to developers and system administrators who deal with YAML configurations, making it an indispensable tool for those frequently working with data in a structured format. It is especially useful for DevOps teams in the context of managing and automating tasks that involve configuration files, enhancing productivity by allowing users to edit YAML files directly from the command line.
Use Case 1: Output a YAML File in Pretty-Print Format (v4+)
Code:
yq eval path/to/file.yaml
Motivation:
When dealing with YAML files, especially those generated or processed by automated systems, the formatting can be less than ideal for human readability. Pretty-printing a YAML file helps in visualizing the structure and data clearly, making it easier to understand and debug the content.
Explanation:
yq
: This is the command-line tool being invoked.eval
: This command reads and processes a YAML file.path/to/file.yaml
: This specifies the path to the YAML file you wish to pretty-print. The file path can be relative or absolute.
Example Output:
name: John Doe
age: 30
contact:
email: john.doe@example.com
phone: 123-456-7890
Use Case 2: Output a YAML File in Pretty-Print Format (v3)
Code:
yq read path/to/file.yaml --colors
Motivation:
For developers using version 3 of yq
, this syntax provides an equivalent output to the pretty-print formatting available in version 4. It ensures consistency in file output presentation across varying versions of the tool.
Explanation:
yq
: Invokes the tool for YAML processing.read
: This command reads a YAML file and processes it for output.path/to/file.yaml
: The location of the YAML file to be processed.--colors
: This argument adds syntax highlighting, enhancing readability.
Example Output:
In color-enhanced terminal environments, output appears similar to v4 pretty-print, though with color distinctions to aid user understanding.
Use Case 3: Output the First Element in a YAML File Containing Only an Array (v4+)
Code:
yq eval '.[0]' path/to/file.yaml
Motivation:
YAML files containing arrays can be unwieldy when you need a quick look at or work with specific elements. Accessing the first element of an array is a common task, whether you’re dealing with configurations, user lists, or any sequential data.
Explanation:
yq
: The tool being used to interact with the YAML data.eval
: Command to process the YAML file.'[0]'
: This path expression targets the first element in an array from the YAML structure.path/to/file.yaml
: File path of the YAML data source.
Example Output:
If the YAML structure is an array of names:
- Alice
- Bob
- Charlie
The output will be:
Alice
Use Case 4: Output the First Element in a YAML File Containing Only an Array (v3)
Code:
yq read path/to/file.yaml '[0]'
Motivation:
Maintaining compatibility with different tool versions is critical, especially in teams with diverse environments. This syntax achieves similar results in version 3, ensuring smooth operations across various setups.
Explanation:
yq
: Command tool for YAML handling.read
: Command to process and read YAML data.path/to/file.yaml
: File path to your YAML data.'[0]'
: Array index specification for extracting first element.
Example Output:
The output for an array:
Alice
Use Case 5: Set (or Overwrite) a Key to a Value in a File (v4+)
Code:
yq eval '.key = "value"' --inplace path/to/file.yaml
Motivation:
Updating configuration files without manual editing accelerates development and deployment processes. Automating key-value updates reduces human error and ensures consistency.
Explanation:
yq
: The YAML processing tool being used.eval
: A command to evaluate expressions and modify YAML data.'.key = "value"'
: Syntax specifying the key and value to set in the YAML.--inplace
: Flag indicating changes should be applied directly to the file.path/to/file.yaml
: File path where the key-value pair is being set.
Example Output:
Upon running the command, the YAML file is modified to contain:
key: value
Use Case 6: Set (or Overwrite) a Key to a Value in a File (v3)
Code:
yq write --inplace path/to/file.yaml 'key' 'value'
Motivation:
For teams that still operate using version 3 of yq
, the ability to programmatically update YAML configurations while minimizing human intervention remains crucial.
Explanation:
yq
: Command for YAML editing.write
: Command option to update or create entries in YAML.--inplace
: Modifier to perform the operation directly on the file.path/to/file.yaml
: The target YAML file.'key' 'value'
: Specifies the key to update and the new value.
Example Output:
The YAML file is updated to include:
key: value
Use Case 7: Merge Two Files and Print to stdout
(v4+)
Code:
yq eval-all 'select(filename == "path/to/file1.yaml") * select(filename == "path/to/file2.yaml")' path/to/file1.yaml path/to/file2.yaml
Motivation:
Data aggregation from multiple YAML files simplifies management and interoperability, especially useful in scenarios where configurations are split or when combining datasets for analysis.
Explanation:
yq
: Command-line tool for handling YAML.eval-all
: Command to process multiple YAML files simultaneously.select(filename == "path/to/file1.yaml") * select(filename == "path/to/file2.yaml")
: Logic to select and multiply data from both files for merging.path/to/file1.yaml path/to/file2.yaml
: Multiple filenames to be merged.
Example Output:
Assuming both YAMLs contain user details, merged content could appear as:
users:
- name: Alice
- name: Bob
Use Case 8: Merge Two Files and Print to stdout
(v3)
Code:
yq merge path/to/file1.yaml path/to/file2.yaml --colors
Motivation:
For version 3 users, the ability to combine content effortlessly without writing to disk aids in visual inspections and seamless integration workflows.
Explanation:
yq
: The YAML processing and merging tool.merge
: Command for aggregating content from multiple YAML files.path/to/file1.yaml path/to/file2.yaml
: Paths to be merged.--colors
: Optional syntax coloring argument for improved readability on output.
Example Output:
The combined content of file 1 and file 2 yields merged YAML data, structured for subsequent processing.
Conclusion:
The yq
command-line tool offers robust capabilities for manipulating YAML files through various versions with flexible syntax options. It’s an essential utility for environments dealing with YAML configuration, enhancing efficiency, and ensuring data consistency across applications and development stages. Whether you require simple visualization or complex data merging, yq
is equipped to meet diverse needs seamlessly.