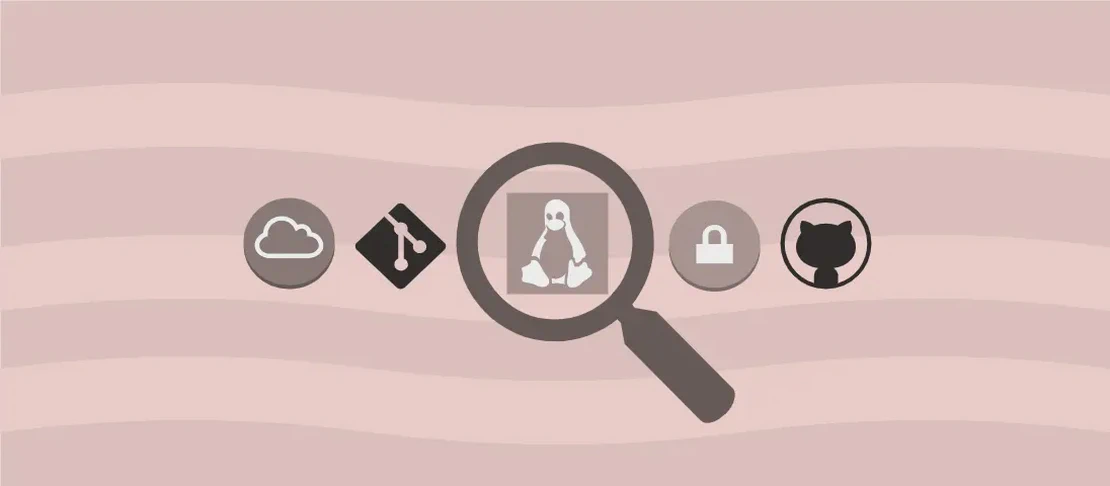
How to use the command 'zek' (with examples)
Zek is a command-line tool that allows you to generate a Go struct from XML. It is a handy tool for developers working with XML data in Go applications, as it automates the process of generating the struct representation of the XML data.
Use case 1: Generate a Go struct from a given XML from stdin
and display output on stdout
Code:
cat path/to/input.xml | zek
Motivation:
The above command is useful when you want to quickly generate a Go struct representation of an XML document and display it on the console. By using cat
to read the XML data from a file and zek
to generate the Go struct, you can easily see the structure of the XML data without having to save it to a file or perform any additional steps.
Explanation:
cat path/to/input.xml
: Reads the XML data from the specified file path and pipes it to thezek
command.zek
: Generates a Go struct from the XML data received fromstdin
and displays the output onstdout
.
Example output:
type Root struct {
XMLName xml.Name `xml:"Root"`
Data string `xml:"Data"`
}
Use case 2: Generate a Go struct from a given XML from stdin
and send output to a file
Code:
curl -s https://url/to/xml | zek -o path/to/output.go
Motivation:
In this use case, you can use zek
along with curl
to download an XML document from a URL and generate the Go struct representation of the XML data. By specifying the -o
option, you can save the generated Go struct to a file for later use or further modification.
Explanation:
curl -s https://url/to/xml
: Downloads the XML data from the specified URL and pipes it to thezek
command.zek -o path/to/output.go
: Generates a Go struct from the XML data received fromstdin
and saves the output to the specified file path.
Example output (path/to/output.go):
package main
import (
"encoding/xml"
"fmt"
"io/ioutil"
"log"
"net/http"
)
type Root struct {
XMLName xml.Name `xml:"Root"`
Data string `xml:"Data"`
}
func main() {
resp, err := http.Get("https://url/to/xml")
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
log.Fatal(err)
}
var r Root
err = xml.Unmarshal(body, &r)
if err != nil {
log.Fatal(err)
}
fmt.Printf("%+v\n", r)
}
Use case 3: Generate an example Go program from a given XML from stdin
and send output to a file
Code:
cat path/to/input.xml | zek -p -o path/to/output.go
Motivation:
In this use case, you can use zek
to not only generate the Go struct representation of the XML data but also a basic Go program that demonstrates how to parse the XML using the generated struct. This can be useful when you want to quickly see the working code that utilizes the generated Go struct.
Explanation:
cat path/to/input.xml
: Reads the XML data from the specified file path and pipes it to thezek
command.zek -p -o path/to/output.go
: Generates the Go struct from the XML data received fromstdin
, along with a basic Go program that demonstrates how to parse the XML using the generated struct, and saves the output to the specified file path.
Example output (path/to/output.go):
package main
import (
"encoding/xml"
"fmt"
"io/ioutil"
"log"
"os"
)
type Root struct {
XMLName xml.Name `xml:"Root"`
Data string `xml:"Data"`
}
func main() {
xmlFile, err := os.Open("path/to/input.xml")
if err != nil {
log.Fatal(err)
}
defer xmlFile.Close()
byteValue, err := ioutil.ReadAll(xmlFile)
if err != nil {
log.Fatal(err)
}
var r Root
xml.Unmarshal(byteValue, &r)
fmt.Printf("%+v\n", r)
}
Conclusion:
The zek
command is a valuable tool for Go developers working with XML data. It simplifies the process of generating a Go struct representation of an XML document, whether it be from a file, URL, or stdin
. The provided use cases demonstrate how to use zek
in various scenarios, from quickly inspecting the structure of an XML document to generating example Go programs that utilize the generated struct.