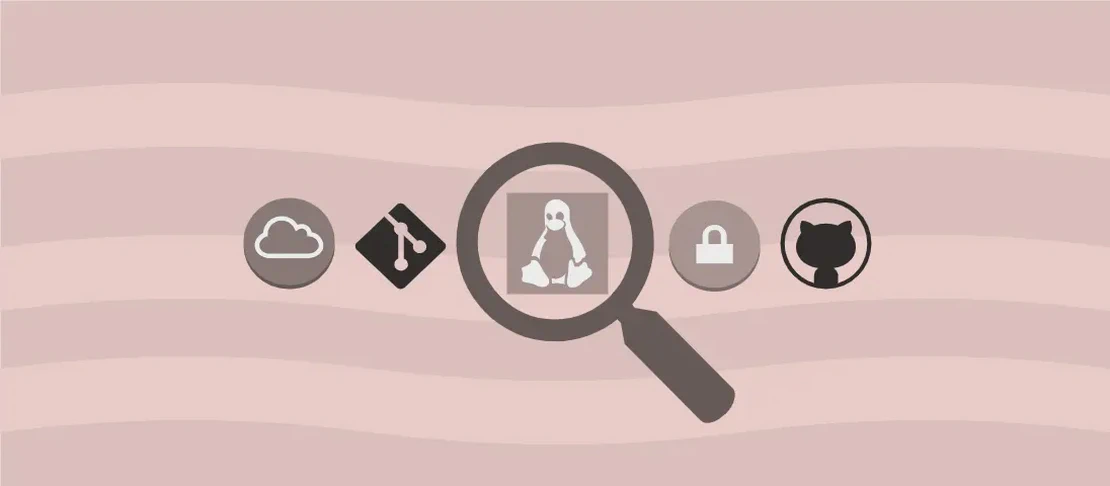
Understanding the Zig Command (with Examples)
Zig is a robust programming language that elevates software development through its focus on simplicity, performance, and safety. Designed to replace C and C++ in various system-level programming applications, Zig offers a modern approach with a compilation and toolchain that is intuitive yet powerful. This article provides detailed use cases demonstrating essential Zig commands, offering clear motivation, explanation, and potential output for each command.
Use Case 1: Compiling a Project in the Current Directory
Code:
zig build
Motivation:
Compiling a project is the fundamental task when working with any programming language. This command condenses all source files and resources into the designated output, preparing it for execution and distribution. Doing this in Zig is particularly beneficial for developers eager to leverage Zig’s cutting-edge optimizations and safety checks without delving deep into configuration complexities.
Explanation:
The zig build
command invokes Zig’s build system, which looks for a build.zig
file in the current directory. This file should define all build rules for the project. The command streamlines the building process, managing dependencies and ensuring that the project is compiled using the correct settings.
Example Output:
Executing this command typically produces binaries (or other specified targets) in a zig-out/bin
directory, depending on the configuration within the build.zig
file. If the build succeeds, you might see:
Zig successfully built in zig-out/bin/
Use Case 2: Compiling and Running the Project
Code:
zig build run
Motivation:
This command steps beyond just compiling; it also executes the project immediately. This is particularly useful for rapid testing and development cycles, where the developer needs to ensure that the integrated project components work harmoniously.
Explanation:
The run
subcommand instructively chains the build process to immediately run the resultant binary. This eliminates the need to manually find the binary and execute it, facilitating a more efficient development workflow focused on immediate feedback and iteration.
Example Output:
When successful, the output window will present messages from the compiler, followed by the runtime output of the initiated executable, such as:
Building...
Running...
Hello, Zig!
Use Case 3: Initializing a Zig Build Project
Code:
zig init
Motivation:
Initialization is crucial when starting new projects, as it lays down a scaffold structure necessary for consistent development. With zig init
, developers can instantly generate starter files and directories, organizing a project at its embryonic stage.
Explanation:
By employing zig init
, the command populates the current directory with a src
folder, a corresponding main.zig
file for the primary executable source, and a foundational build.zig
configuration script. These serve as a template for developing either a library or executable with Zig.
Example Output:
Upon execution, the directory structure is formed:
.
├── build.zig
└── src
└── main.zig
Initialized empty Zig project
Use Case 4: Creating and Running a Test Build
Code:
zig test path/to/file.zig
Motivation:
Testing is an integral part of ensuring software reliability and quality. Zig treats testing as a first-class citizen, encompassing the same level of importance as writing the application logic itself.
Explanation:
The zig test
command compiles and executes test declarations within the specified source file (path/to/file.zig
). As part of Zig’s dedication to safety, this process helps identify bugs and logical errors by executing code in an isolated environment.
Example Output:
Upon success or failure, Zig details the results of all tests within the specified source file:
1/1 tests passed.
or
Test failure in path/to/file.zig at line 23.
Assertion failed!
Use Case 5: Cross-Compiling and Running for a Different Architecture and OS
Code:
zig build run -fwine -Dtarget=x86_64-windows
Motivation:
Cross-compilation becomes indispensable when developers need to target different environments without needing the native build environment. This Zig command supports building projects for another OS or architecture directly from a single development workstation, broadening the range of application compatibility.
Explanation:
-fwine
: This flag suggests the use of Wine to run Windows binaries on Unix-like systems, key for easing cross-platform testing.-Dtarget=x86_64-windows
: Specifies the target architecture and OS for cross-compilation, dictating the tailored compilation of executables compatible with the specified environment.
Example Output:
The resultant command might produce:
Cross compiled successfully to x86_64-windows.
Running under Wine...
Hello from a Windows executable!
Use Case 6: Reformatting Zig Source Code
Code:
zig fmt path/to/file.zig
Motivation:
Consistent code formatting is foundational in maintaining readability and code quality across teams and projects. Zig’s formatting tool ensures uniform style application, reducing stylistic inconsistencies.
Explanation:
The fmt
subcommand systematically reformats the specified Zig source file (path/to/file.zig
) into its canonical form, providing a universally recognizable structured view of the code.
Example Output:
Running this might return no direct screen output, as it’s intended to modify the file in place:
The `path/to/file.zig` has been reformatted.
Use Case 7: Translating a C File to Zig
Code:
zig translate-c -lc path/to/file.c
Motivation:
Bridging between C and Zig, this command assists developers in migrating existing C codebases to Zig, facilitating the exploration of Zig’s improved safety and concurrency features.
Explanation:
translate-c
: This directs Zig to reinterpret C code into equivalent Zig code.-lc
: Links the standard C library, ensuring that standard C functions are available in the translated code for resolving dependencies.
Example Output:
Generated output Zig file might look like:
// Example of translated header and function
const std = @import("std");
export fn myFunction() void {
// body translation
}
Use Case 8: Using Zig as a Drop-in C++ Compiler
Code:
zig c++ path/to/file.cpp
Motivation:
This use case illustrates Zig’s flexibility, as it seamlessly interacts with other established languages like C++. Here, Zig becomes a compatible C++ compiler replacing traditional options.
Explanation:
The c++
subcommand specifies compilation of a C++ file using Zig’s tooling infrastructure, thus aligning the compatibility and modern advantages of Zig’s compiler capabilities with C++ development.
Example Output:
Running it can yield the compiled C++ output, much like invoking a standard C++ compiler:
Compiled successfully with Zig.
Conclusion
The Zig command-line tool embodies a comprehensive suite of commands that simplify diverse aspects of system-level programming, including compiling projects, testing, cross-compiling, code formatting, and code translation. Zig incorporates modern programming paradigms, facilitating efficient software development across various environments by elevating safety, performance, and readability. By mastering these commands, developers can optimize their workflow while leveraging the full potential of Zig as a contemporary system programming language.