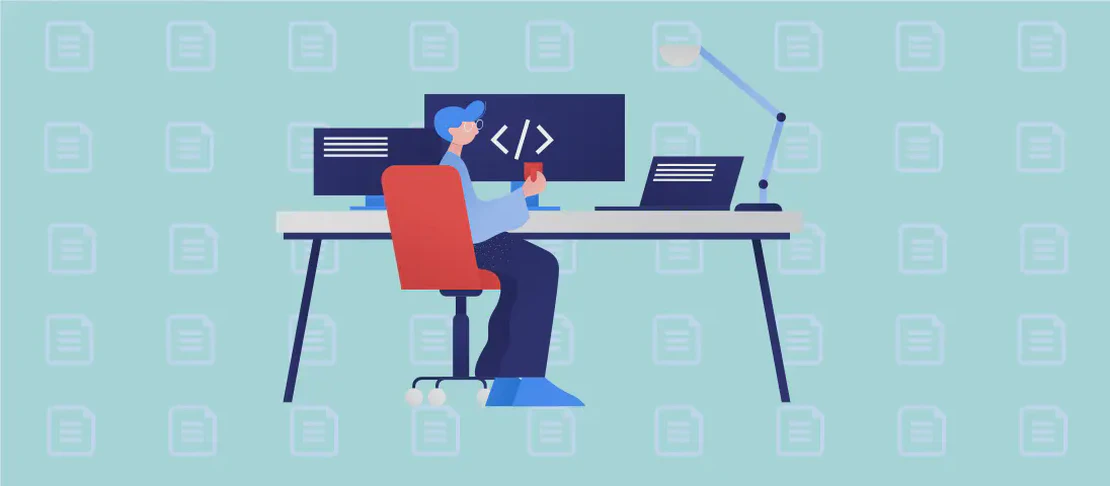
How to Use the Command 'zip' (with Examples)
The ‘zip’ command is a powerful utility in the UNIX and Linux environments used for packaging and compressing files into a Zip archive. This tool is essential for reducing file sizes for storage efficiency and convenient file transfer over networks. It can also secure files by encrypting them with a password. This article outlines various use cases of the ‘zip’ command, providing detailed explanations and motivations for each.
Use Case 1: Add Files/Directories to a Specific Archive Recursively
Code:
zip -r path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Archiving files and directories recursively is essential when you want to compress an entire directory, including all subdirectories and files contained within them. This is particularly useful when backing up a project folder or compressing a website’s directory structure for deployment.
Explanation:
zip
: Invokes the zip command.-r
: Stands for recursive, which means that the command will include all files and subdirectories within the directories specified.path/to/compressed.zip
: Specifies the destination path and name for the resultant compressed archive.path/to/file_or_directory1 path/to/file_or_directory2 ...
: Lists one or more files or directories to be added into the archive.
Example Output:
Creating archive path/to/compressed.zip adding:
- path/to/file_or_directory1
- path/to/file_or_directory2
Use Case 2: Remove Files/Directories from a Specific Archive
Code:
zip -d path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Occasionally, after creating a zip archive, you might realize that certain files or directories should not have been included. Instead of recreating the archive from scratch, you can use this command to delete unwanted items efficiently.
Explanation:
zip
: Initiates the Zip command process.-d
: Specifies delete, instructing the command to remove the files or directories listed from the archive.path/to/compressed.zip
: This is the archive from which files or directories will be removed.path/to/file_or_directory1 path/to/file_or_directory2 ...
: The specific files or directories that you want to delete from the archive.
Example Output:
deleting: path/to/file_or_directory1
deleting: path/to/file_or_directory2
Use Case 3: Archive Files/Directories Excluding Specified Ones
Code:
zip -r path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ... -x path/to/excluded_files_or_directories
Motivation: Sometimes, you may need to archive a directory but exclude certain large files or directories that are not required, such as temporary files, logs, or development files. This command allows you to do just that.
Explanation:
zip
: The command to start archiving.-r
: Indicates that the operation should be recursive, including all subdirectories unless excluded.path/to/compressed.zip
: The location and name of the destination compressed archive.path/to/file_or_directory1 path/to/file_or_directory2 ...
: Directories or files to be archived, except those specified in-x
.-x
: This argument specifies exceptions, i.e., files or directories to be excluded from the archive.
Example Output:
Including all except:
- path/to/excluded_files_or_directories
Use Case 4: Archive Files/Directories with a Specific Compression Level
Code:
zip -r -0..9 path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Adjusting the compression level allows you to control the trade-off between the size of the archive and the time required to create it. Higher compression levels produce smaller files but can be significantly slower to process, and vice versa.
Explanation:
zip
: Command initiator.-r
: Recursive, meaning the whole directory and its contents are compressed.-0..9
: Represents the compression level.0
is no compression,9
is maximum compression.path/to/compressed.zip
: The path where the compressed archive will be created.path/to/file_or_directory1 path/to/file_or_directory2 ...
: The files or directories to be included in the archive.
Example Output:
Compression level set to: 9
Creating archive path/to/compressed.zip
Use Case 5: Create an Encrypted Archive with a Specific Password
Code:
zip -r -e path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Security is vital when transferring sensitive files. Encrypting a zip file protects its contents with a password, ensuring that only authorized personnel can access the information.
Explanation:
zip
: Starts the zipping process.-r
: Recursively archives files and directories.-e
: Enables encryption of the archive, requiring a password for extraction.path/to/compressed.zip
: Designated path and file name for the encrypted zip archive.path/to/file_or_directory1 path/to/file_or_directory2 ...
: Files or directories to be encrypted and added to the archive.
Example Output:
Enter password:
Verify password:
Use Case 6: Archive Files/Directories to a Multi-part Split Zip Archive
Code:
zip -r -s 3g path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Handling large files can be problematic, especially when there are size restrictions for transfer or storage. Splitting archives into smaller parts makes them more manageable and easier to distribute across different storage media or platforms.
Explanation:
zip
: Invokes the Zip command.-r
: Indicates recursive archiving.-s
: Set the size of each split part of the archive;3g
specifies 3 gigabytes per part.path/to/compressed.zip
: Destination path for the multi-part archive.path/to/file_or_directory1 path/to/file_or_directory2 ...
: Source directories or files for archiving.
Example Output:
Creating parts:
Creating part: path/to/compressed.z01 (3 GB)
Creating part: path/to/compressed.z02 (3 GB)
Creating part: path/to/compressed.zip (Remaining)
Use Case 7: Print a Specific Archive’s Contents
Code:
zip -sf path/to/compressed.zip
Motivation: Before extracting or managing a zip archive, it’s often crucial to verify its contents. This command lets you list all files and directories in the archive without extracting them, ensuring you handle it appropriately.
Explanation:
zip
: This command prefix is necessary to perform the operation.-sf
: Lists the contents of the specified archive.path/to/compressed.zip
: The archive whose contents you wish to display.
Example Output:
Listing contents of path/to/compressed.zip:
- file_1.txt
- directory_1/
- directory_1/file_2.txt
Conclusion
The ‘zip’ command is a versatile tool in any user’s toolkit, offering solutions for compressing, organizing, and securing data in Linux and UNIX systems. Whether creating backups, preparing files for network transfer, or securing sensitive data, knowing how to utilize these various use cases of the ‘zip’ command can greatly enhance productivity and efficiency.