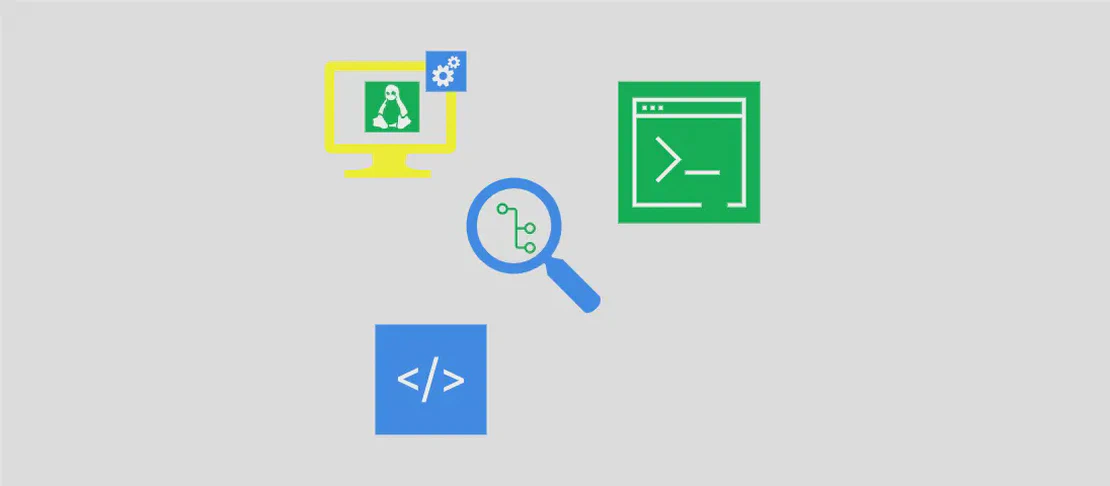
How to Use the 'zip' Command (with examples)
- Linux
- December 17, 2024
The zip
command is a powerful utility used for packaging and compressing multiple files or directories into a single archived file with the .zip
extension. It is commonly used for reducing the disk space required by files and for simplifying their distribution. The zip
command provides several functionalities, allowing users to add or remove files from an archive, set specific compression levels, encrypt archives, create multi-part archives for large files, and view contents of an archive. Understanding how to leverage each of its capabilities efficiently is essential for effective file management and transfer.
Use case 1: Add files/directories to a specific archive
Code:
zip -r path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Creating a compressed archive that contains multiple files or directories is an efficient way to organize and store related files together. An archive not only helps in saving disk space through compression but also makes it easier to distribute a single file rather than multiple files.
Explanation:
zip
: The command used to create a new archive or update an existing one.-r
: This option ensures that the operation is recursive, meaning it includes all files and directories within any directories specified.path/to/compressed.zip
: The destination file path where the archive will be created. It should have the.zip
extension.path/to/file_or_directory1
,path/to/file_or_directory2
: These are the files or directories you wish to add to the archive.
Example Output: Creating archive ‘path/to/compressed.zip’ and adding specified files/directories.
adding: path/to/file_or_directory1/ (stored 0%)
adding: path/to/file_or_directory2/ (deflated 92%)
Use case 2: Remove files/directories from a specific archive
Code:
zip --delete path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Sometimes you need to update an archive by removing outdated or unnecessary files or directories. This method provides a way to manage the contents of an existing archive without having to recreate it from scratch.
Explanation:
zip
: The basic command to handle zipped files.--delete
: Specifies that the designated files and directories should be removed from the archive.path/to/compressed.zip
: Indicates the archive from which the files or directories will be removed.path/to/file_or_directory1
,path/to/file_or_directory2
: The files or directories that should be deleted from the archive.
Example Output: Updating the archive by removing specified files/directories.
deleting: path/to/file_or_directory1/
deleting: path/to/file_or_directory2/
Use case 3: Archive files/directories excluding specified ones
Code:
zip path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ... --exclude path/to/excluded_files_or_directories
Motivation: When creating an archive, there may be files or directories within your selection that you do not want to include due to size, redundancy, or privacy concerns. This option allows you to selectively avoid adding such content to the archive.
Explanation:
zip
: Initiates the command to perform a zip operation.path/to/compressed.zip
: The destination file for the new archive.path/to/file_or_directory1
,path/to/file_or_directory2
: Specifies what is to be included in the archive.--exclude path/to/excluded_files_or_directories
: Designates file(s) or directories that should be omitted from the archive.
Example Output: Creating an archive while excluding specified files/directories.
adding: path/to/file_or_directory1/ (deflated 20%)
adding: path/to/file_or_directory2/ (deflated 45%)
excluding: path/to/excluded_files_or_directories
Use case 4: Archive files/directories with a specific compression level
Code:
zip -r -9 path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Compression levels affect both the size of the resulting archive and the time required to create it. When dealing with limited storage capacity or bandwidth, using the highest compression level can significantly reduce the file size, albeit potentially at the cost of increased time to compress.
Explanation:
zip
: Command to compress files into a zip format.-r
: Specifies recursive operation to include all nested files and directories.-9
: Indicates the highest level of compression, aiming to achieve the smallest possible file size.path/to/compressed.zip
: The output file path for the archive.path/to/file_or_directory1
,path/to/file_or_directory2
: Designates the content to be archived with high compression.
Example Output: Creating a highly compressed archive.
adding: path/to/file_or_directory1/ (deflated 82%)
adding: path/to/file_or_directory2/ (deflated 90%)
Use case 5: Create an encrypted archive with a specific password
Code:
zip -r --encrypt path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: Security concerns could necessitate the encryption of sensitive information within an archive. An encrypted zip file requires a password before the contents can be accessed, ensuring that the data remains confidential and protected during storage and transmission.
Explanation:
zip
: The command used to execute the creation of the archive.-r
: Encompasses all files within the specified directories recursively.--encrypt
: Triggers the encryption process for the archive, prompting for a password.path/to/compressed.zip
: The targeted zip archive that will be encrypted.path/to/file_or_directory1
,path/to/file_or_directory2
: Files and directories to be encrypted in the archive.
Example Output: Prompt for password protection during archive creation.
adding: path/to/file_or_directory1/ (deflated 18%)
Enter password:
Verify password:
adding: path/to/file_or_directory2/ (deflated 35%)
Use case 6: Archive files/directories to a multi-part split Zip archive
Code:
zip -r -s 3g path/to/compressed.zip path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation: When dealing with very large files, or in cases where the storage medium has size limitations (such as USB drives or DVDs), creating a multi-part zip archive can be indispensable. Splitting the archive into fixed-size parts ensures that each piece can be handled individually, facilitating storage and transfer across constrained environments.
Explanation:
zip
: Executes the command to archive files.-r
: Specifies recursion, including nested files and directories.-s 3g
: Splits the archive into parts with a maximum size of 3 gigabytes each.path/to/compressed.zip
: The resultant multi-part archive base name.path/to/file_or_directory1
,path/to/file_or_directory2
: Contents targeted for inclusion in the segmented archive.
Example Output: Archiving into multiple parts based on specified size.
splitting: path/to/compressed.zip.z01 (3 GB)
splitting: path/to/compressed.zip.z02 (3 GB)
adding: path/to/file_or_directory1/ (deflated 61%)
adding: path/to/file_or_directory2/ (deflated 70%)
Use case 7: Print a specific archive contents
Code:
zip -sf path/to/compressed.zip
Motivation: Before extracting or sending a zip file, it can be useful to review the list of files within it to ensure that it contains the expected data or to verify its contents without needing to decompress the entire archive. This command offers a quick inspection of the archive’s contents.
Explanation:
zip
: The command acting upon the zip archive.-sf
: Displays a short file list for the archive.path/to/compressed.zip
: The archive file whose contents are to be listed.
Example Output: Overview of archive contents.
Archive contains:
path/to/file_or_directory1/
path/to/file_or_directory2/
total 2 entries (0.01% compression)
Conclusion:
Understanding the zip
command’s diverse functionality helps effectively manage file storage, secure sensitive information, and streamline file distribution. Each use case provides unique ways to customize archive handling, which can be important across different contexts such as conserving disk space, facilitating data transmission, or ensuring data privacy. Mastery of these zip
capabilities transforms file and directory management tasks into efficient and secure operations.