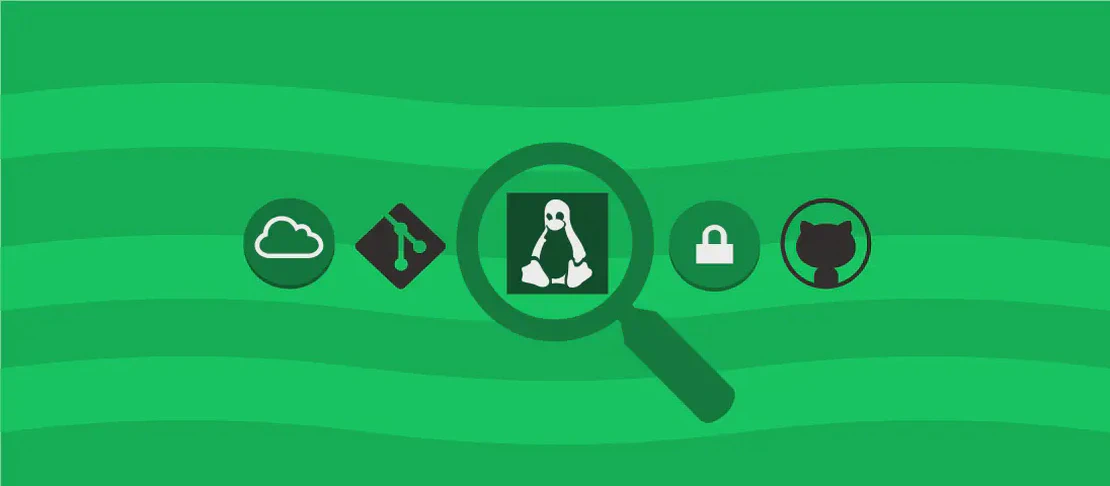
How to Use the Command 'zsh' (with examples)
Z SHell (zsh) is an interactive shell and command-line interpreter that is an extended version of the Bourne Shell (sh) with plenty of new features and support for plugins and themes. Designed for efficiency and user-friendly scripting, zsh is both powerful and flexible, making it a preferred choice for developers and power users who require a robust shell environment.
Use case 1: Start an Interactive Shell Session
Code:
zsh
Motivation: Starting an interactive shell session with zsh is essential when you want to leverage its advanced features and functionalities in real-time. Whether you’re developing scripts, executing commands, or just navigating your filesystem, an interactive session provides an environment for on-the-fly command execution and experimentation.
Explanation:
The command zsh
with no arguments initiates an interactive shell session. Once the shell is launched, you can type and execute commands, access command history, and utilize auto-completions that zsh offers.
Example Output: As a result, your terminal prompt will change, indicating that you are currently in a zsh shell environment.
Use case 2: Execute Specific Commands
Code:
zsh -c "echo Hello world"
Motivation: There are scenarios where you want to execute specific commands without starting an interactive shell session. Using the ‘-c’ option suits automated scripts and tasks, such as scheduled cron jobs or remote shell access, where executing single commands is necessary.
Explanation:
The -c
option allows you to pass a command or a series of command-line instructions (’echo Hello world’ in this case) to the zsh for execution and then exit. It’s a straightforward way to run a command directly without interactive engagement.
Example Output:
Hello world
Use case 3: Execute a Specific Script
Code:
zsh path/to/script.zsh
Motivation: Executing a script using zsh is essential when you have scripts specifically written to leverage zsh’s syntax and features. Running scripts allows for executing a sequence of commands stored in a file, providing automation and reusability.
Explanation:
When you specify the path to a script file, such as script.zsh
, zsh reads and executes every command in the script. This use case is crucial for routine tasks or complex operations wrapped into scripts.
Example Output: Assuming the script contains:
echo "Welcome to zsh"
The output will be:
Welcome to zsh
Use case 4: Check a Specific Script for Syntax Errors Without Executing
Code:
zsh --no-exec path/to/script.zsh
Motivation: Before running a script, it’s prudent to check for syntax errors to ensure it executes smoothly. Syntax checking helps identify issues that could lead to script failure, ensuring code reliability and stability.
Explanation:
The --no-exec
flag forces zsh to perform a syntax check on the specified script without actually running its contents. This allows developers to catch errors early in the development process.
Example Output: If there are no syntax errors, there will be no output. For scripts with errors, zsh will notify you with an error message pointing out the problematic line or syntax.
Use case 5: Execute Specific Commands from stdin
Code:
echo Hello world | zsh
Motivation: Piping commands into zsh is useful when you want to pass output from one program directly as input to zsh. This form of command execution is common in UNIX-like operating systems, enabling powerful one-liners.
Explanation:
Here, echo Hello world
sends its output through a pipe to zsh, which then executes it. This is a method to execute commands on the fly without them being embedded in a script file.
Example Output:
Hello world
Use case 6: Execute a Specific Script, Printing Each Command in the Script Before Executing It
Code:
zsh --xtrace path/to/script.zsh
Motivation:
Using the --xtrace
option is valuable for debugging scripts. It provides transparency on what commands are being executed and in what order, which is crucial for diagnosing issues in complex scripts.
Explanation:
The --xtrace
flag enables a mode where each command in the script is printed to standard output before execution. This step-by-step display helps in understanding the script’s flow and identifying potential issues.
Example Output: Assuming the script contains:
echo "Start Script"
echo "End Script"
The output will be:
+echo Start Script
Start Script
+echo End Script
End Script
Use case 7: Start an Interactive Shell Session in Verbose Mode, Printing Each Command Before Executing It
Code:
zsh --verbose
Motivation: Starting zsh in verbose mode allows you to see each command before it executes in the current session. This is particularly helpful for debugging session-specific configurations and understanding shell behavior.
Explanation:
The --verbose
option makes zsh output each command to the terminal before it’s run, providing clearer insight into operations within the shell.
Example Output: With commands executed in this mode, each step will be printed in a verbose manner, although no predefined example exists without user interaction.
Use case 8: Execute a Specific Command Inside zsh with Disabled Glob Patterns
Code:
noglob echo *.txt
Motivation: There are times when you want to prevent globbing, or wildcard expansion, especially if you want to treat wildcards as literal strings and not file patterns. Useful in scripts or commands where glob patterns may have significant meanings or for display purposes.
Explanation:
The noglob
command tells zsh to run the following command (echo *.txt
here) with globbing disabled, treating *.txt
literally rather than trying to match files.
Example Output:
*.txt
Conclusion:
The various use cases of the zsh command demonstrate its versatility and power as a command-line interpreter. Whether you need to execute simple commands, debug scripts, or manage complex shell interactions, zsh offers a robust environment tailored to advanced user requirements. By understanding these examples, you’ll be better equipped to leverage zsh for your computing tasks efficiently.